LET, CONST and VAR
let and const were introduced in ES6. We use let when we want to
allow a variable to change its value in the future, we use const
when we don't want the same (immutable variable).
Const variables cannot be initialized undeclared.
By default, we should use const.
A variable declared with var in the global scope is added as a
property to the window object. The same is NOT true with const or
let.
Variables declared with var are function-scoped, while variables
declared with let or const are block-scoped.
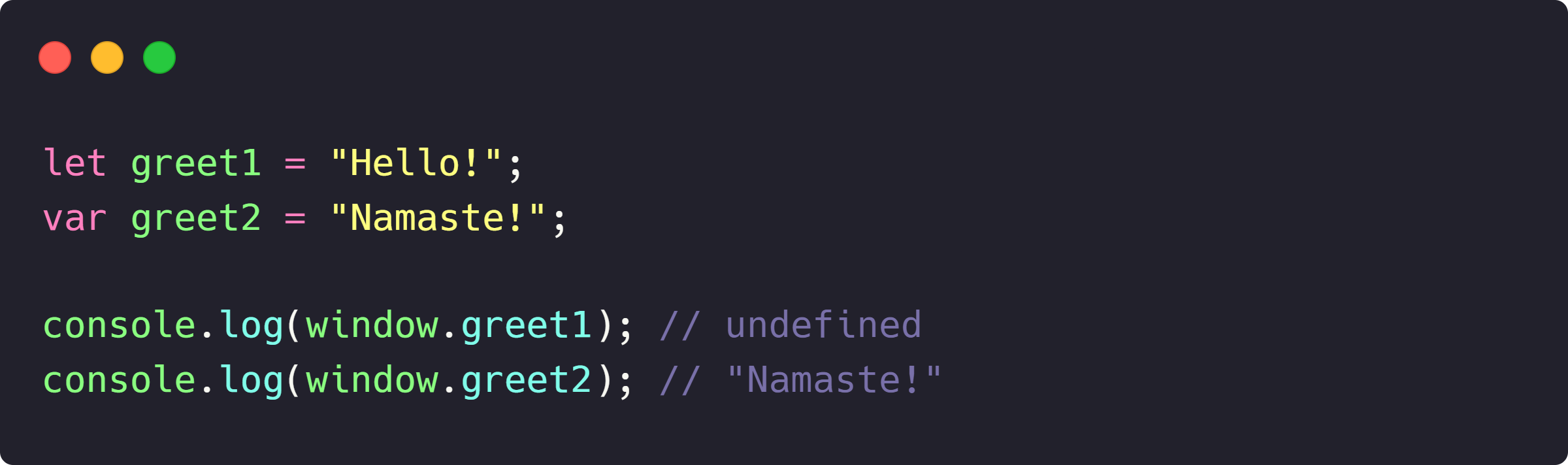
STRINGS AND TEMPLATE LITERALS
We can use backticks `` to declare strings anywhere.
Using ``,
creates a newline when we start writing on a new line.
We can
do any JS inside the curly braces using
${}.
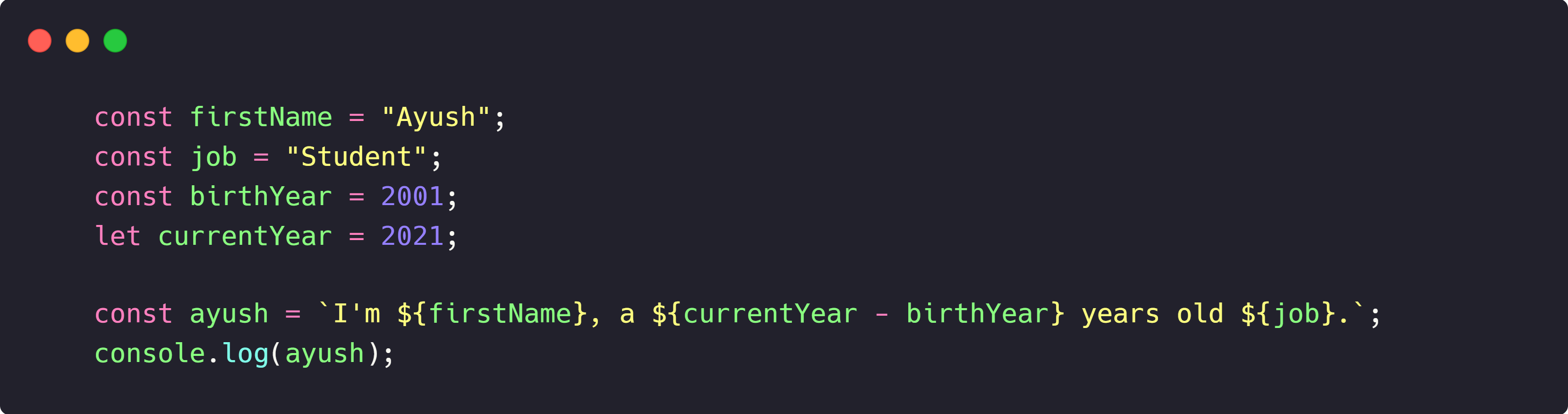
TYPE CONVERSION AND COERCION
Type conversion - When we manually convert a data type.
Type
coercion - When JavaScript does it automatically for us.
To convert any string/number to boolean, we can use Boolean()
function.
To convert a number to a string, use String() function.
To convert a string to a number, use Number() function. Example -

Examples of type coercion -



NOTE - Only + operator coverts numbers to strings, every other mathematical or logical operator converts strings to number by type coercion.
TRUTHY AND FALSY VALUES
Falsy values - These are the values that
when converted to boolean outputs false. They are
1) 0
2) Empty string ('')
3) undefined
4) null
5)
NaN
Truthy values - Apart from the above
values, all the other values output truth when converted to boolean.
USING STRICT MODE
To activate strict mode, we have to write ("use strict";) at the top
of the JS file.
It prevents us from creating new variables when
we mis-type a variable name and therefore create a new one, by
throwing an error.
FUNCTION DECLARATIONS VS. EXPRESSIONS AND ARROW FUNCTIONS
There are 3 ways to define a function, they are -
1) Function declaration
- In this way, we can first call a function and then later define it
in the code below. Example -
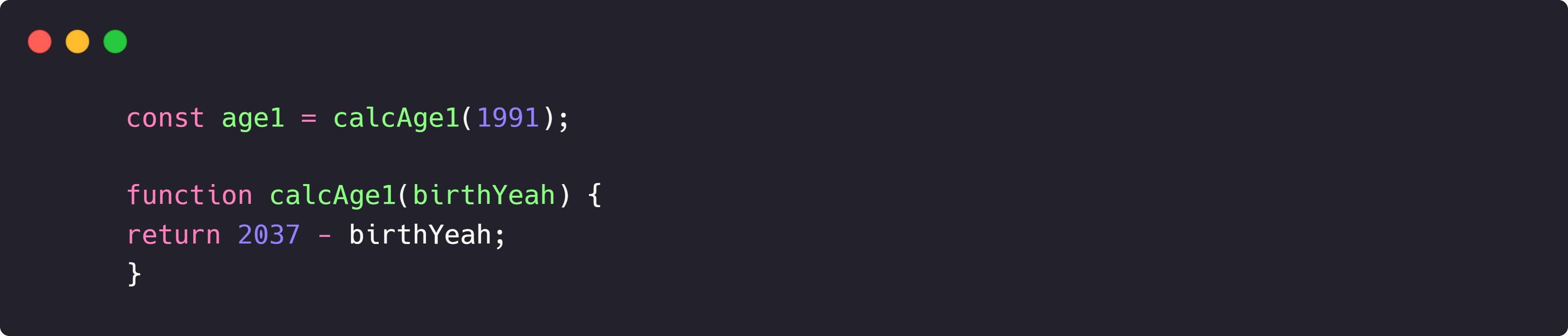
2) Function expression - In this way, we CANNOT first call a function and then later define it in the code below. Example -
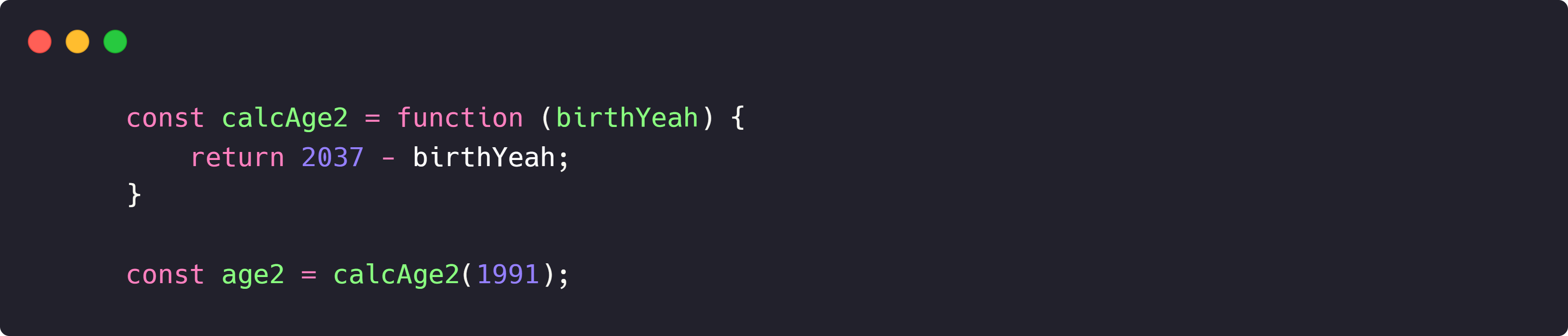
3) Arrow functions - This way is best for
writing one line functions.
Example 1 - This example code has
no parenthesis for parameter and no return word.

Example 2 -
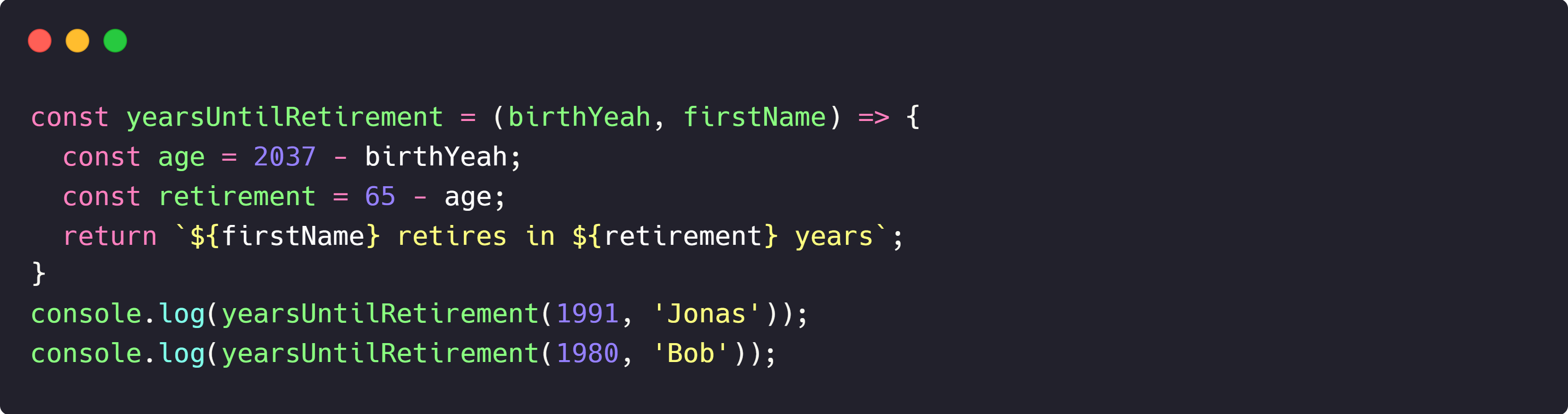
BASIC ARRAY OPERATIONS (METHODS)
1) arrayName.push(newArrayElement) - This
method adds an element at the end of the array. This method returns
the new length of the array.
2) arrayName.unshift(newArrayElement)
- This method adds an element at the start of the array. This method
returns the new length of the array.
3) arrayName.pop()
- This method removes an element from the end of the array. This
method returns the removed element of the array.
4) arrayName.shift()
- This method removes an element from the start of the array. This
method returns the removed element of the array.
5) arrayName.indexOf(arrayElement)
- This method returns the index of the array element that we type
in. It returns -1 if that element does not exists in the array.
6) arrayName.includes(arrayElement) - This
method returns true or false depending that element exists in the
array or not.
JS OBJECTS
When we try to access a property that does not exists, we get
undefined as return value.
Difference between dot and bracket
notation -
NOTE - We use bracket notation whenever we want to
use a variable name to refer to a property.
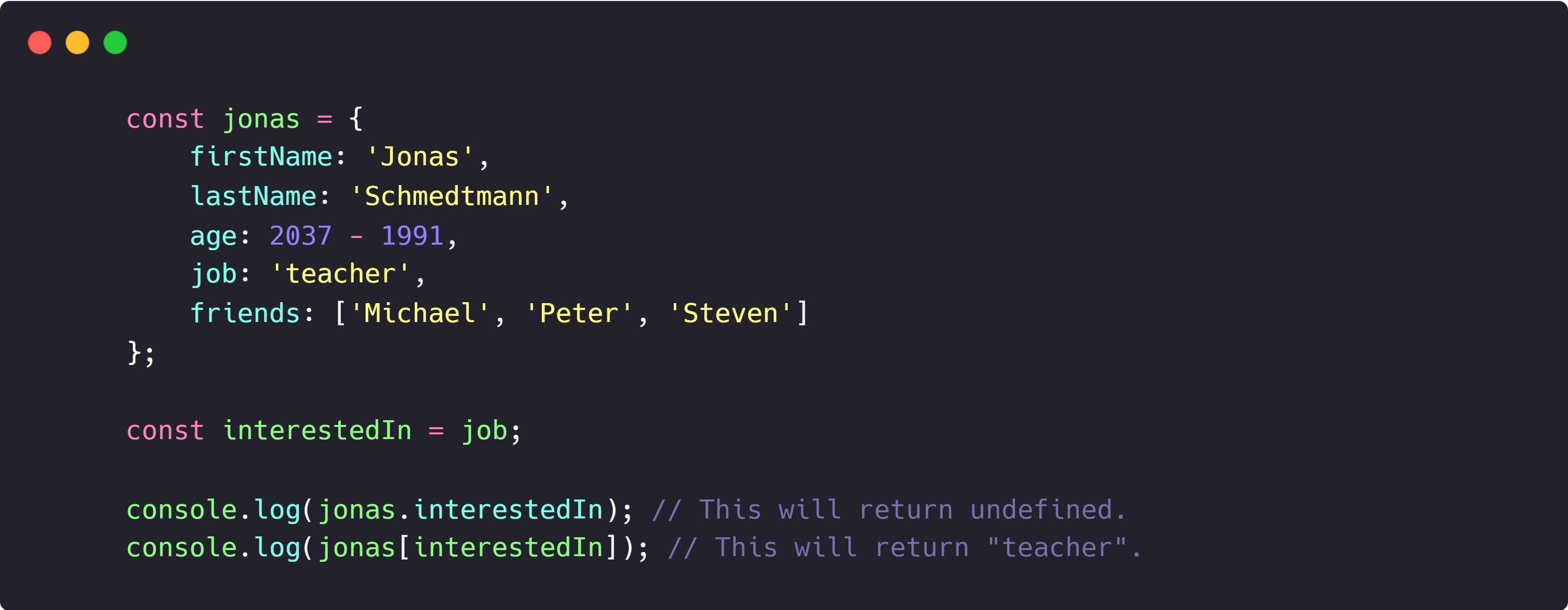
We can even store functions in objects because function expressions
are just values.
Object methods are just functions, that are defined inside the
object. Example -
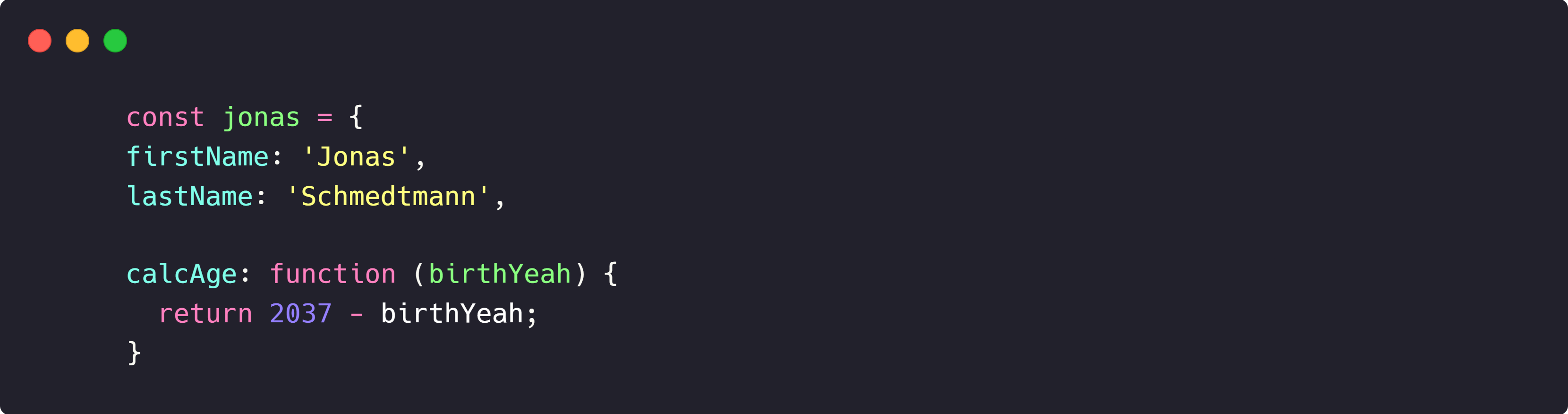
The best practice to call an object method is to call it once and
then store it's result in a variable.
Example -
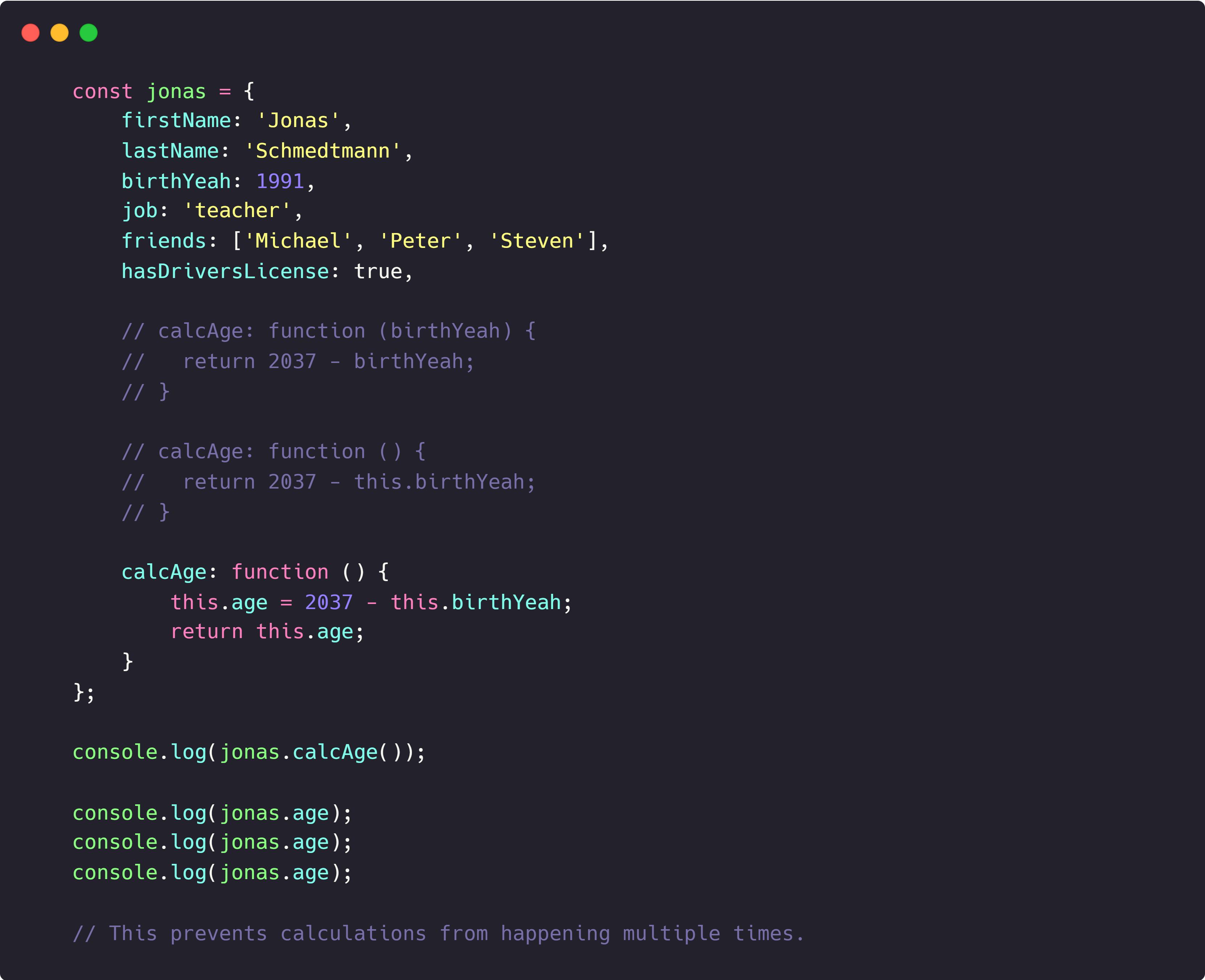