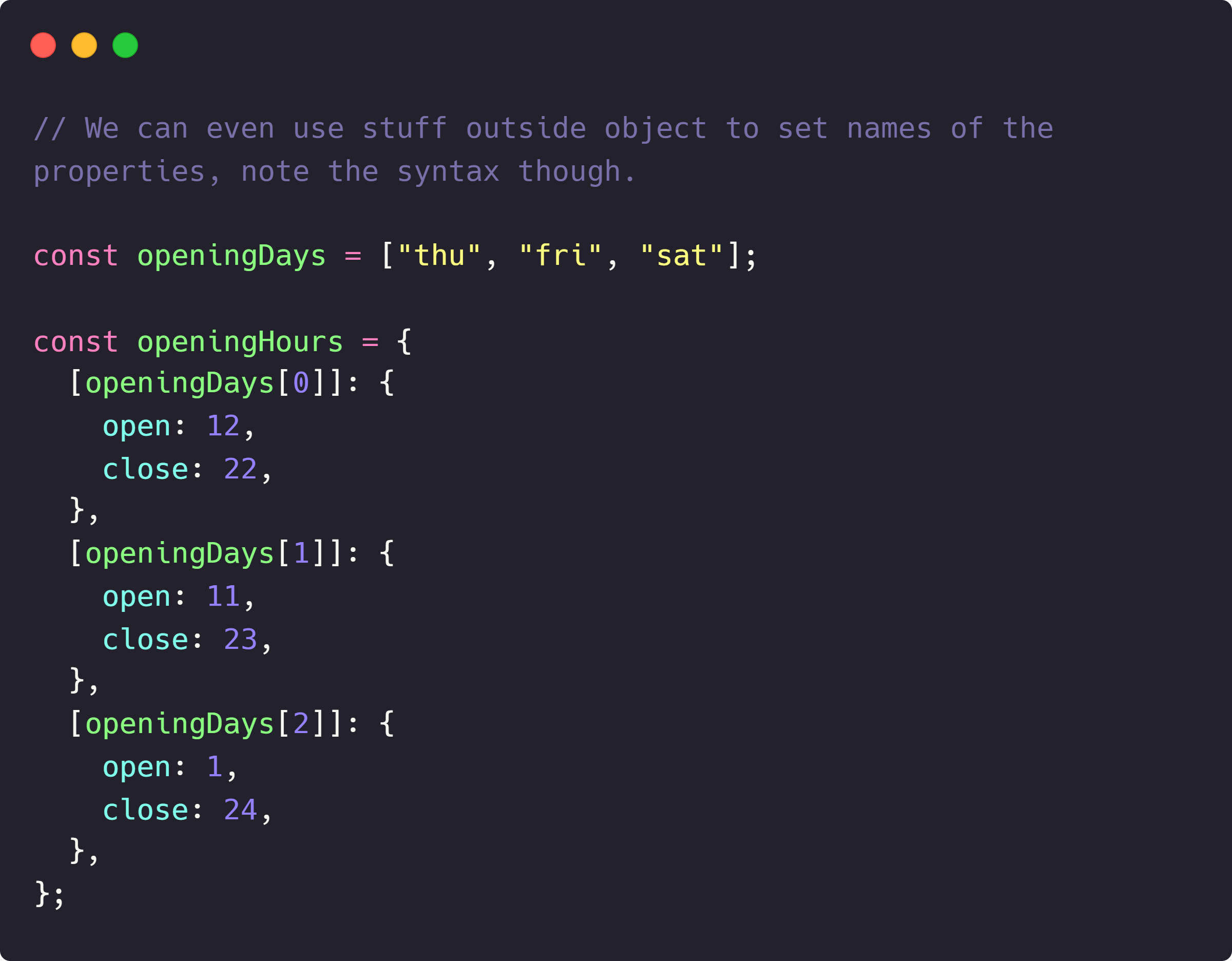
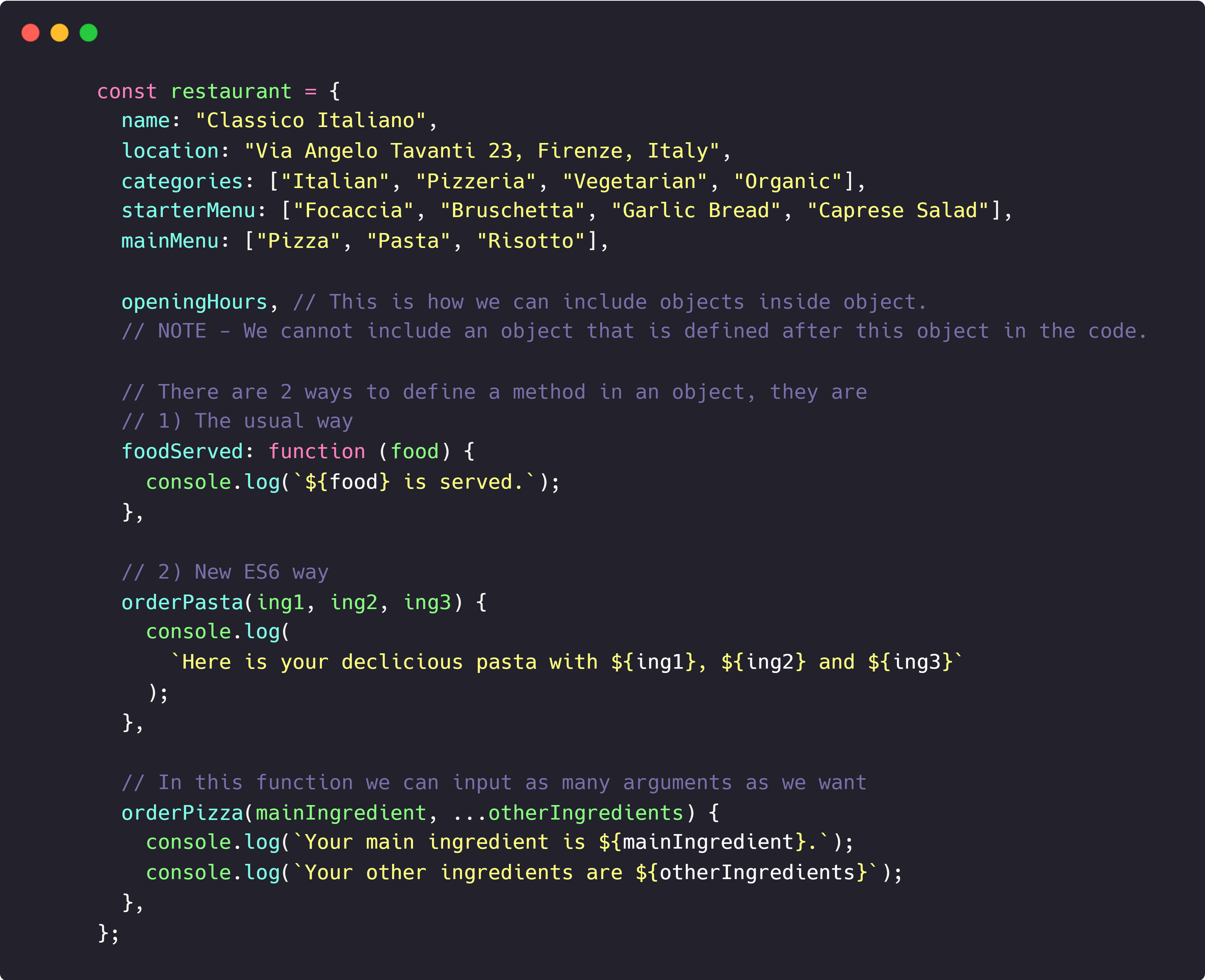
DESTRUCTING ARRAYS
It means to covert the elements of arrays into individual variables.
Example -

To switch the values of 2 variables, we can again use destructing
arrays. This is called mutating variables.
Example -

Nested destructing - Example

We can also set the variables to default value. Example -

DESTRUCTING OBJECTS
Same as destructing arrays.
Exaple -

NOTE - Here property name should be same as property name in the object from which we are copying values.
Nested objects destructing -

THE SPREAD OPERATOR "..."
It spreads the values of an array separately. Example -

To copy an array -

To concat two arrays -

Example of how to use spread operator to give multiple inputs to a function.
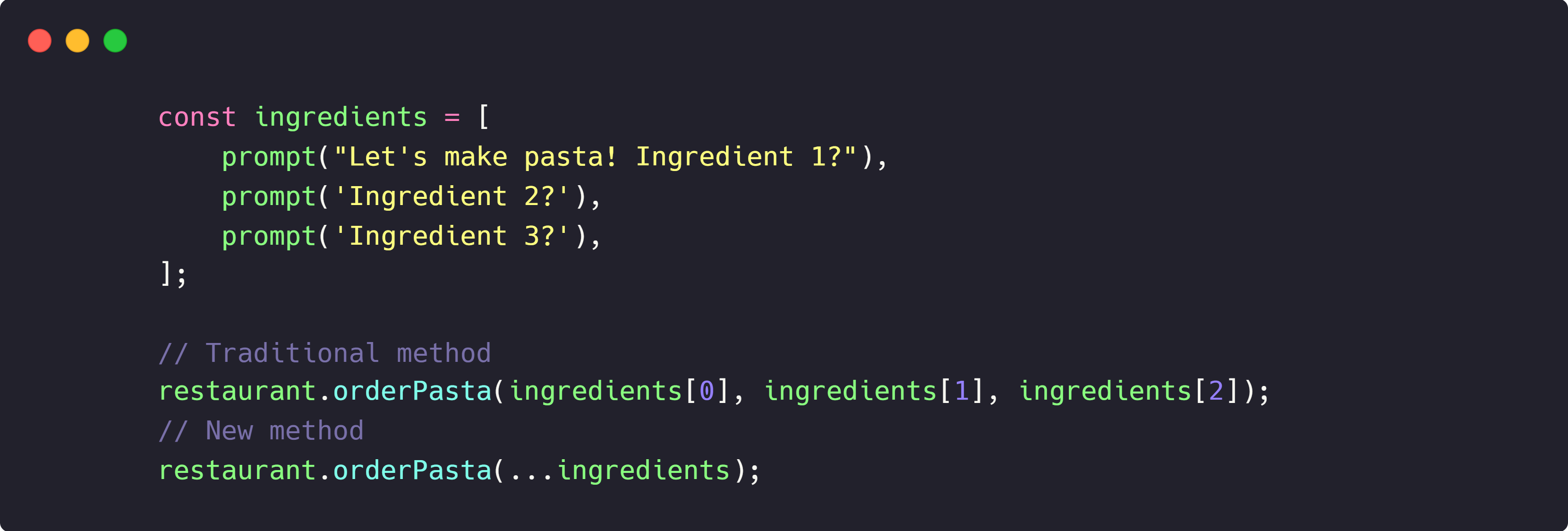
Using spread operator to make a new object -

If the property with the same name already exists in the object that we are spreading, then the property inside the "spreading" object gets prioritized.
THE REST OPERATOR "..."
It has the same syntax as the spread operator, but it does the
opposite of it.
It packs the separate variables into an array.
Examples -
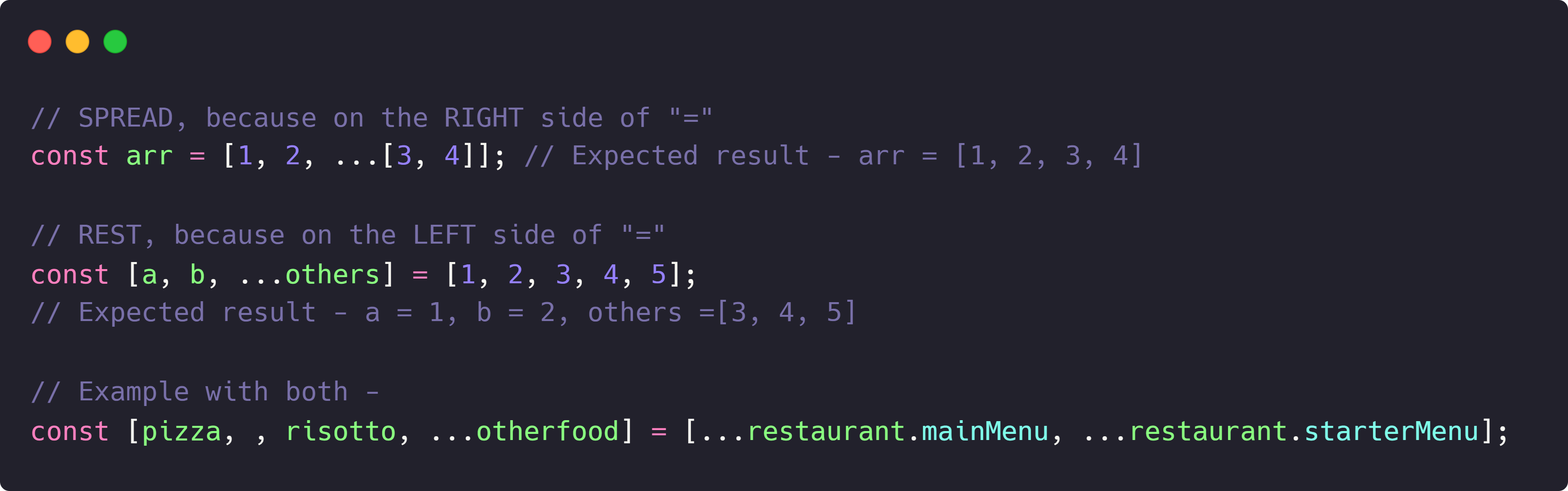
Using rest operator to create objects - Same as arrays, it creates an object since we are using curly braces. Example -

Using rest operator in functions - Here we pass numbers separated by a comma, even though the function expects only a single input. The rest operator here, converts the separate numbers into an array. This method is more preferred because now we can input separate numbers as well as arrays.
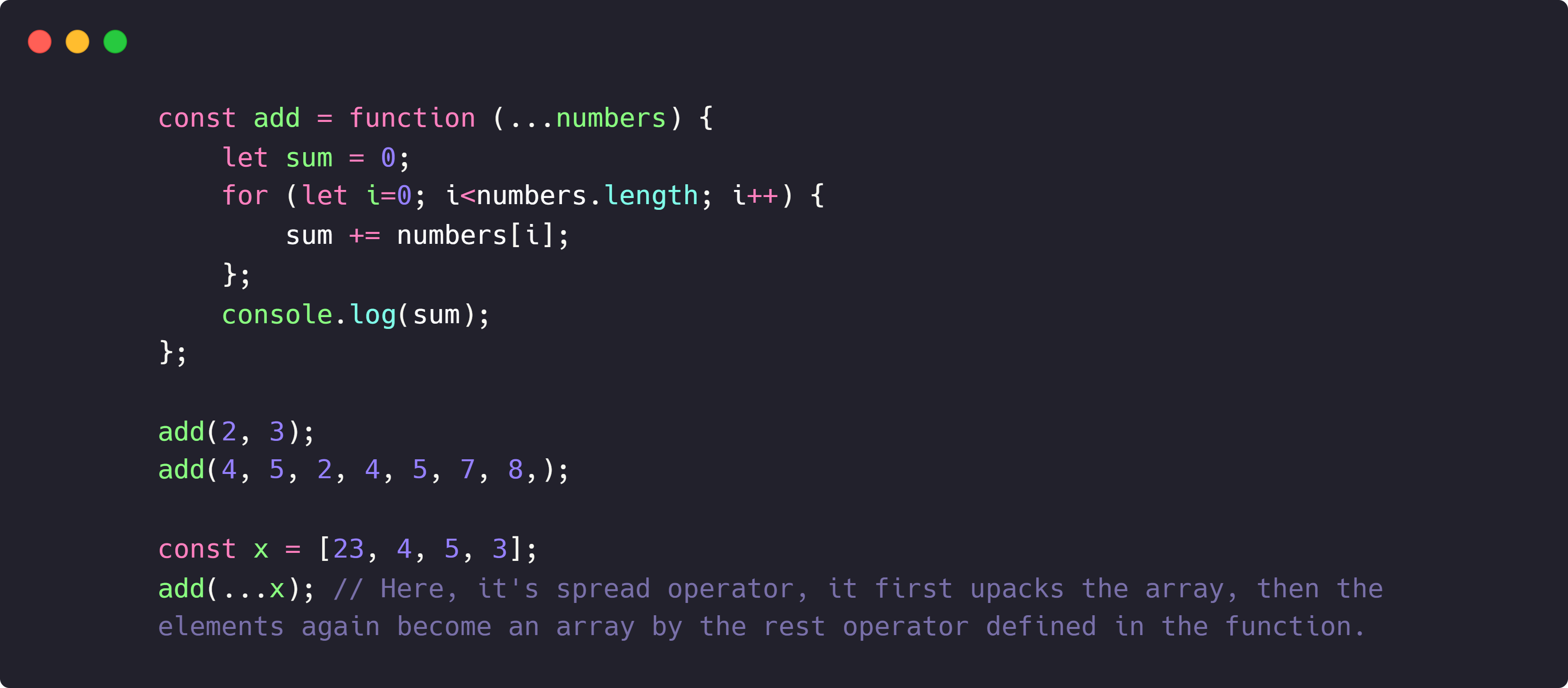
SHORT CIRCUITING (&& AND ||)
|| operator -
It is the same as
logical OR operator. It will check the first value, if that's true,
it will return that value, if it's false, it will move to the next
value. It will continue doing this until a truthy value is reached.
If all the values are falsey, it will return the last value.
This returns the first truthy value or if all the values
are falsey, it will return the last falsey value.
NOTE - Logical operators Use ANY data type, return ANY data
type, follows short-circuiting.
EXAMPLES -
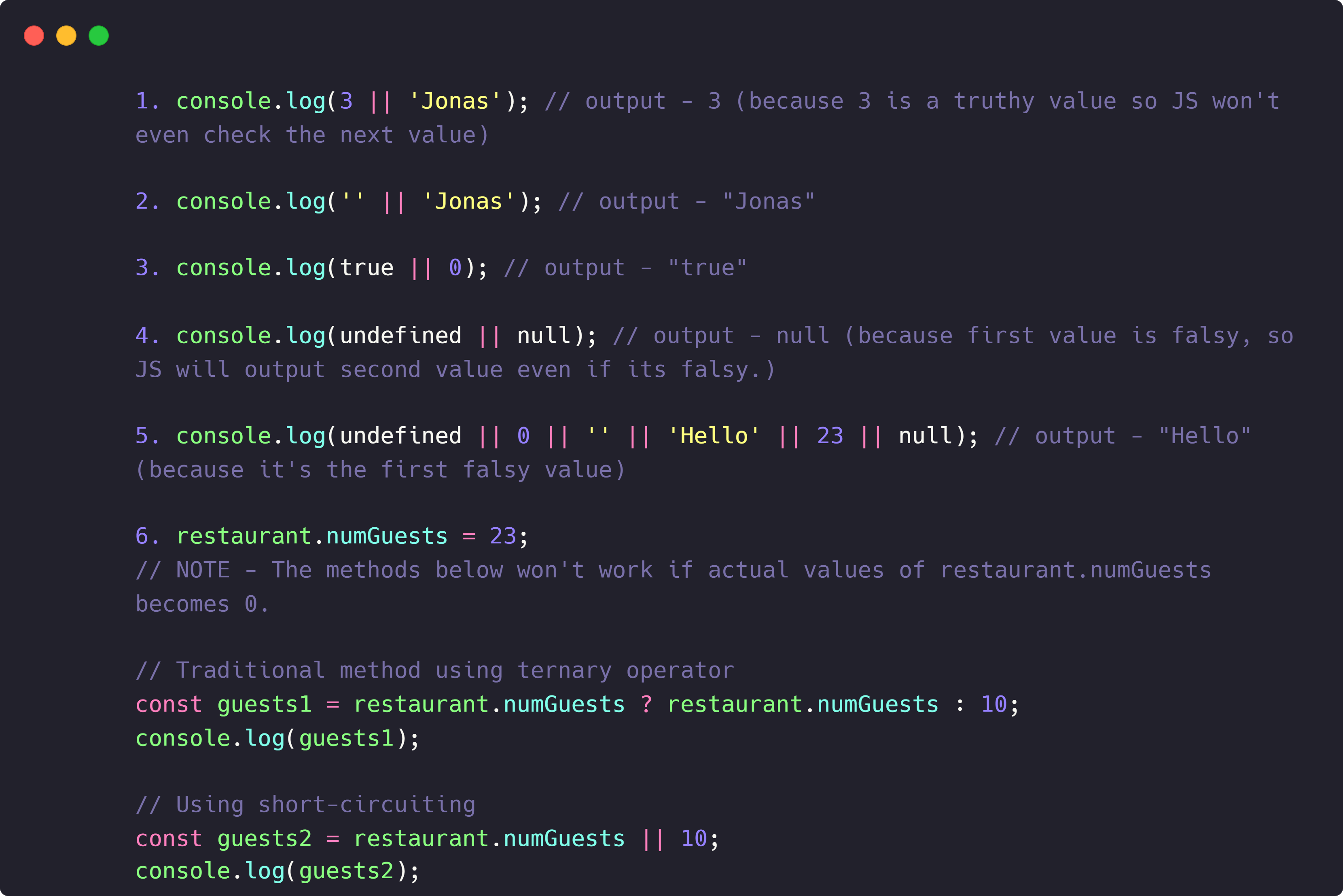
Nullish Coalescing operator ?? -
It is same as || operator, only diff is that, this operator short
circuits based on Nullish values instead of falsey values.
NOTE - Nullish values are null and undefined only (not 0 or
"").
EXAMPLE -
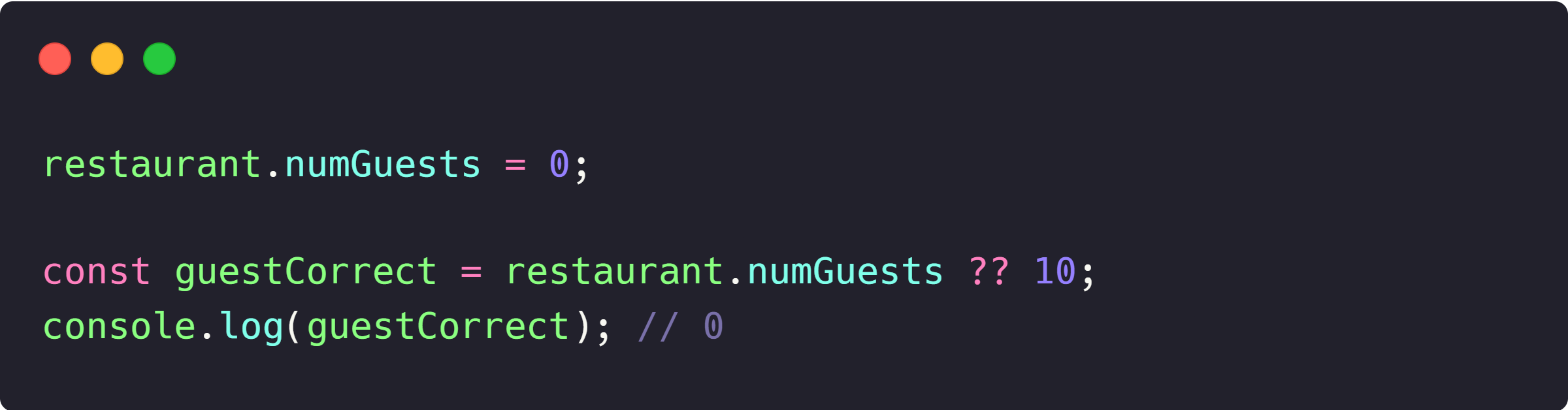
&& operator -
This returns the
first falsey value or if all the values are truthy, it will return
the last truthy value.
EXAMPLES -
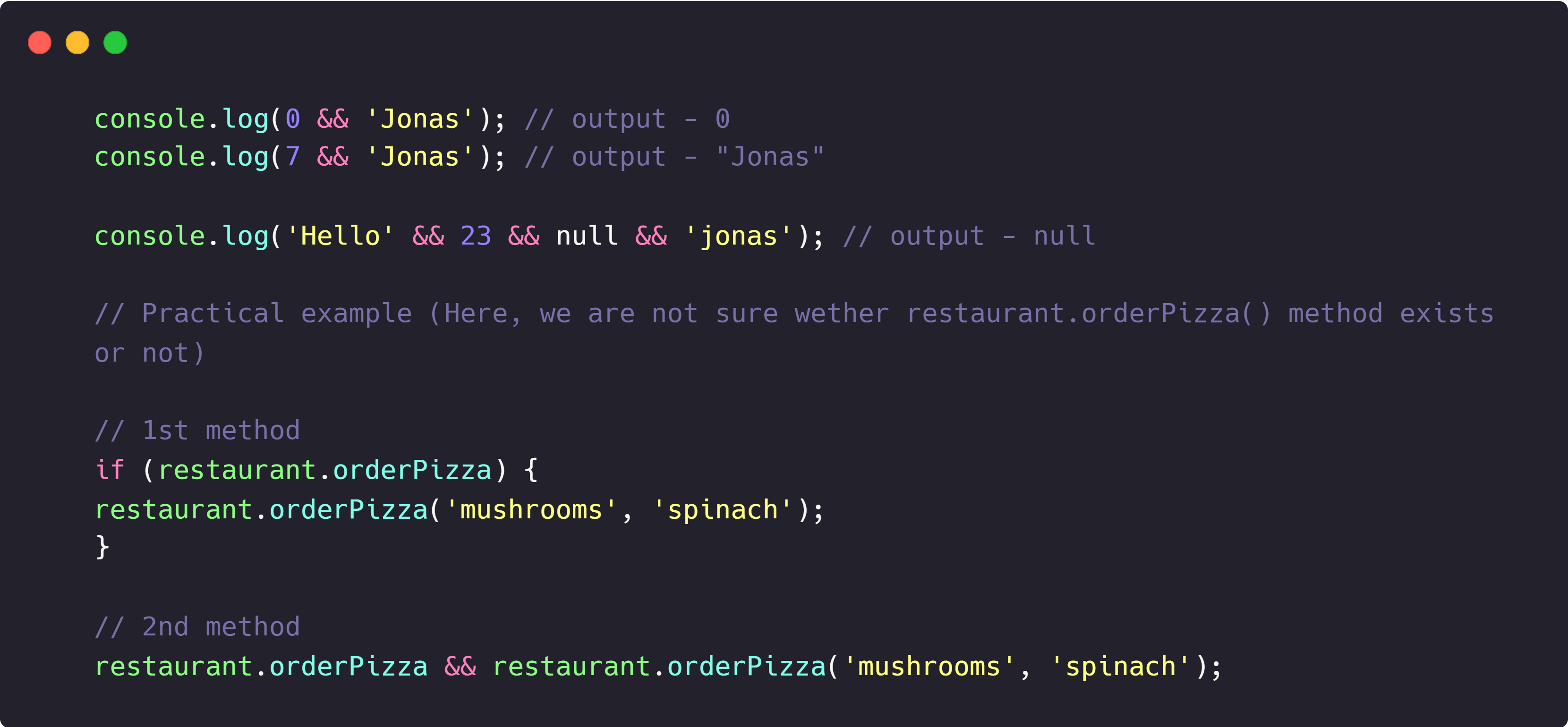
THE FOR OF LOOP
Examples -
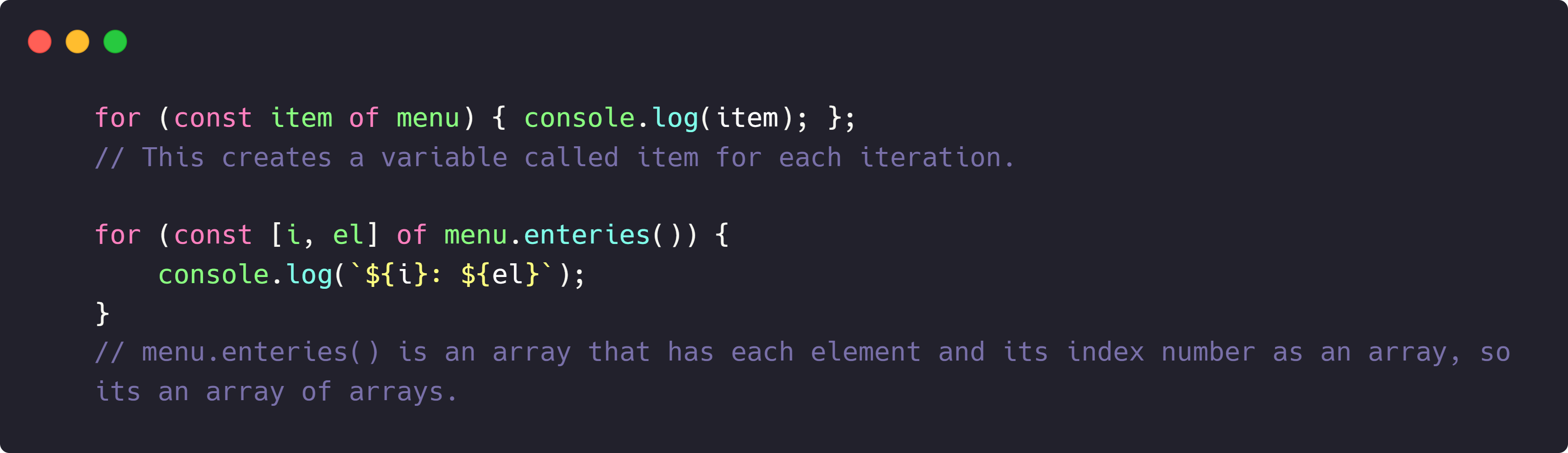
OPTIONAL CHAINING (FEATURE OF ES2020)
It is used for chaining nested objects. It checks wether the
property written before "?" exists or not.
Example -
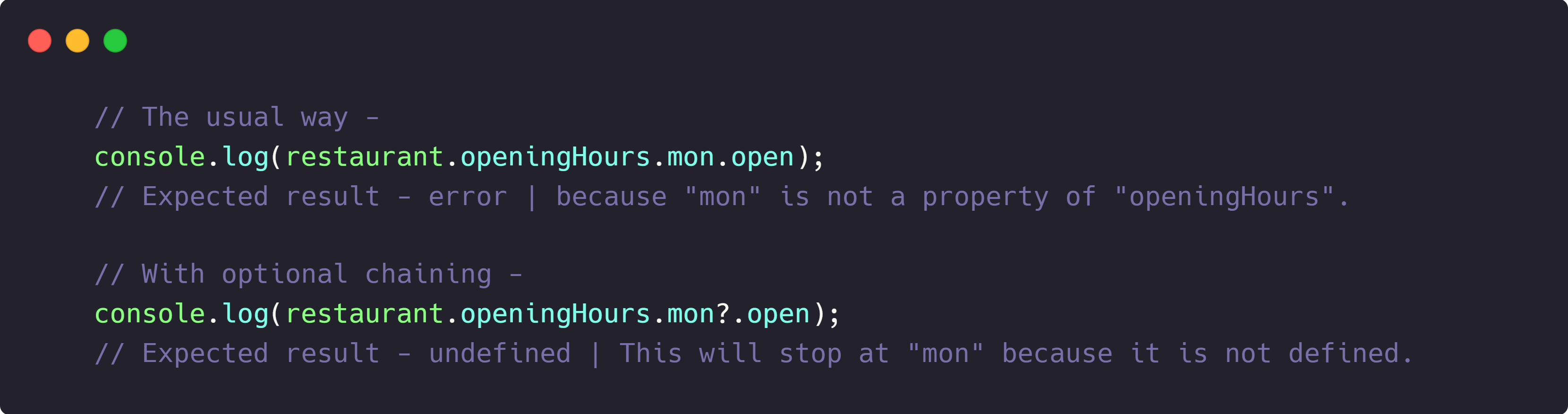
Another Example -
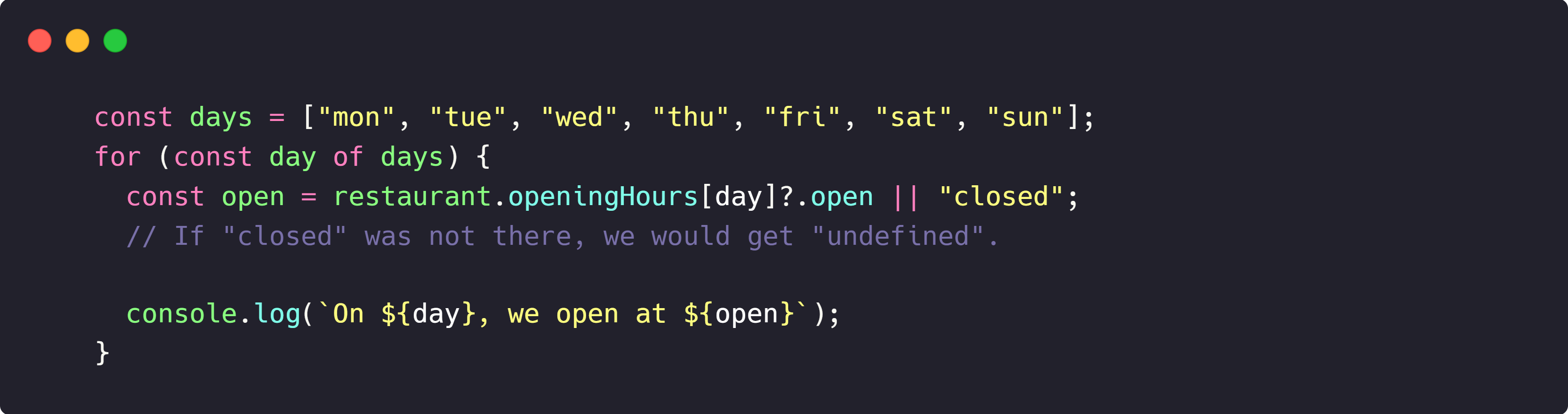
Optional Chaining also works for methods. Here it comes b/w the
method name and parameters.
Example -

Optional chaining also works for arrays. It basically checks if an array is empty.
Example -

LOOPING OVER OBJECTS (OBJECT.KEYS(), OBEJCT.VALUES(), OBJECT.ENTRIES())
We can even loop over objects.
Property NAMES
- This will create an array of property names.
Example -
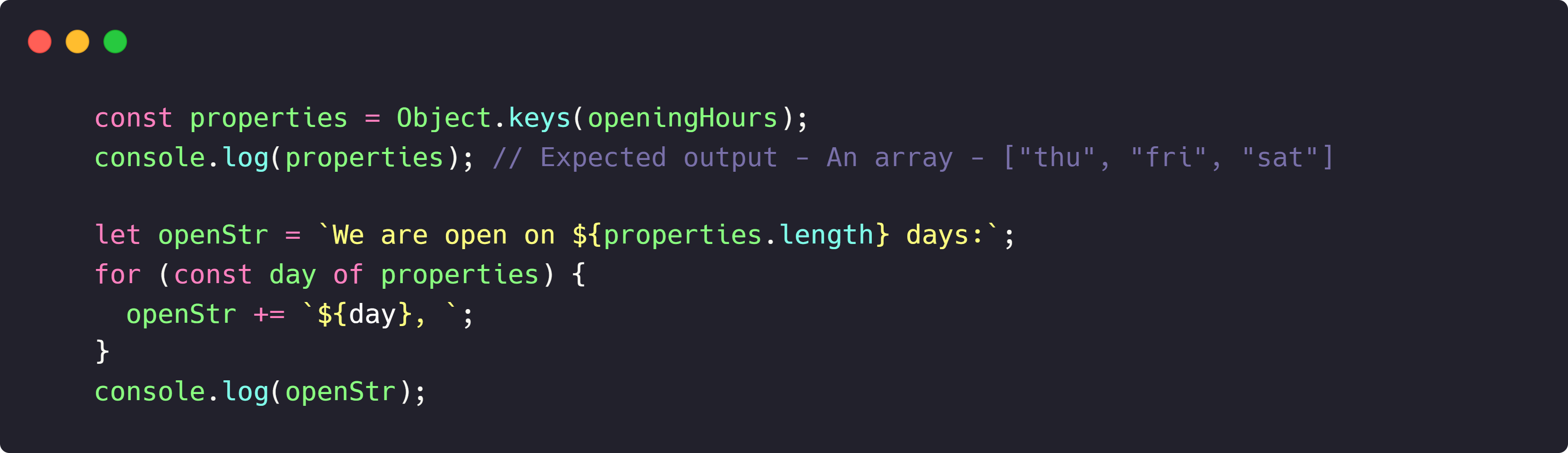
Property VALUES - This will create an array
of property values.
Example -

Entire object - This will create an array,
with each element being a key-value pair.
Example -
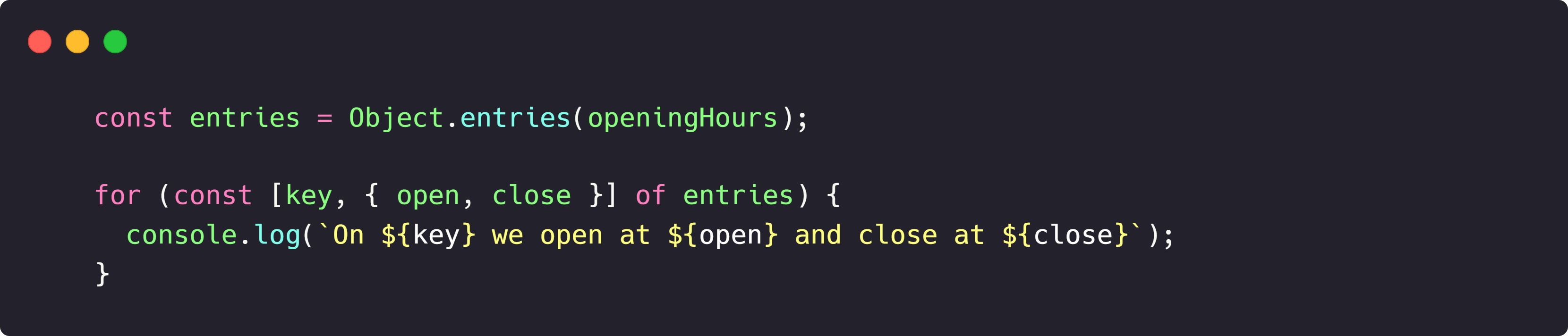
SETS
It is another data structure that only stores unique values.
Example -
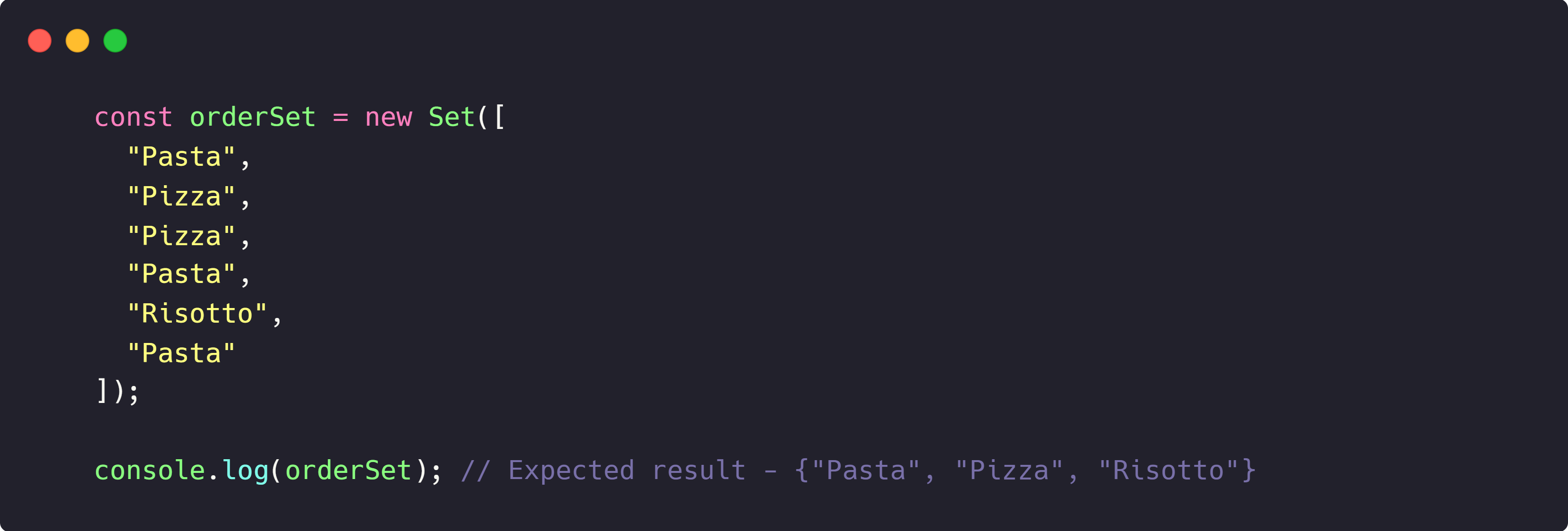
They can be used for all iterables.
Example -

There length can be calculated using the "size" method.
It can also be checked if the set includes some element using "has"
method.
Example -

We can add elements to sets using "add" method, and we can remove
elements using "delete" method.
We can convert a set to an array -

MAPS
Maps are also a data structure that works similar to objects apart
from the fact that keys can be anything in maps whereas in objects,
they can only be strings.
Example -

To add an entry to map, we use set() property.
Example -
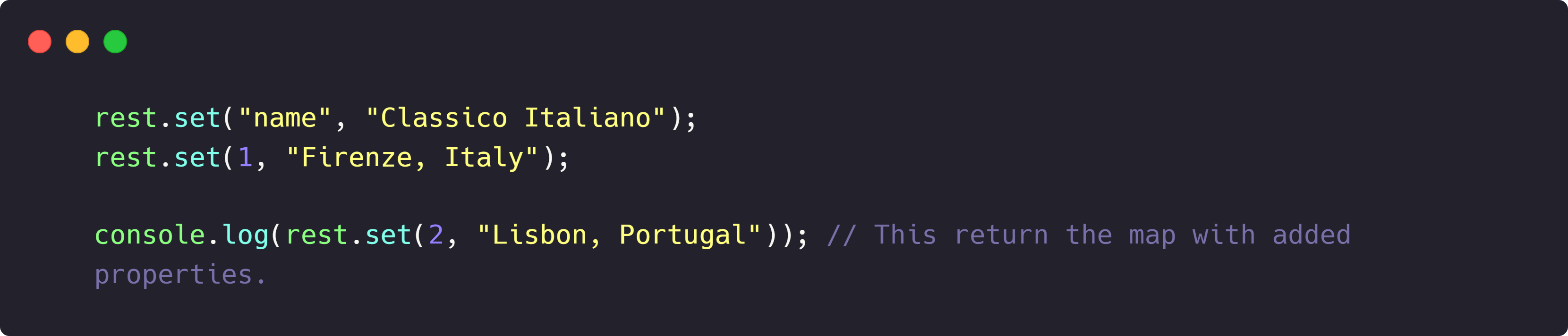
We can even chain the set() properties.
Example -
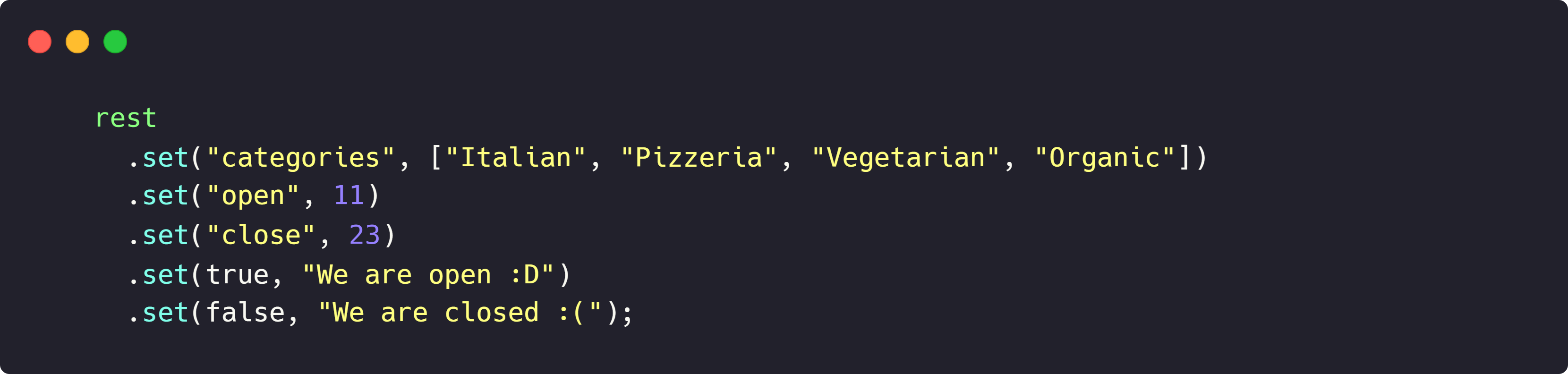

We can delete entries using delete() property. It also has has() and size() method.
STRINGS
Examples -
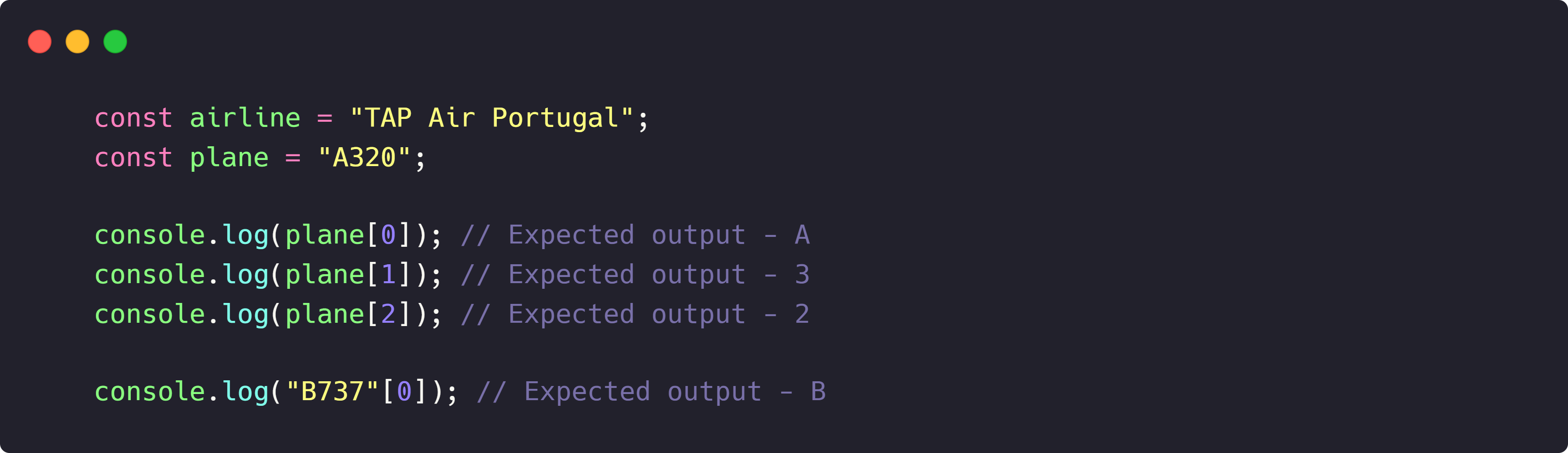
length method

indexOf() method

lastIndexOf() method - This gives the index of last occurence

slice() method -
slice(startingIndex, endingIndex)
If we only give
startingIndex, it will extract the whole string starting from
startingIndex.
startingIndex is included and endingIndex is
excluded.
Length of new string = endingIndex - startingIndex
Examples -

We can also use negative index, which starts from end.
NOTE -
Last character has the index = -1
Examples -

Changing case -
.toLowerCase()
.toUpperCase()
Example - Convert "AyuSH" to "AYush"
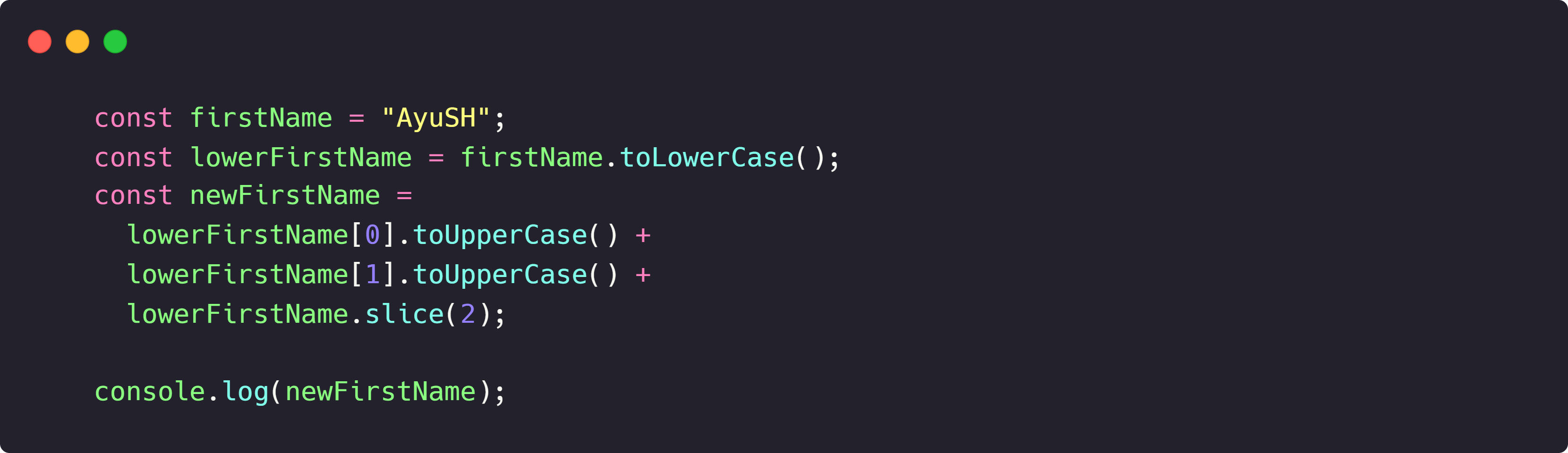
Removing white-spaces -
.trim()
Replacing strings -
.replace("part of
string to remove", "new string to be added their")
Example -
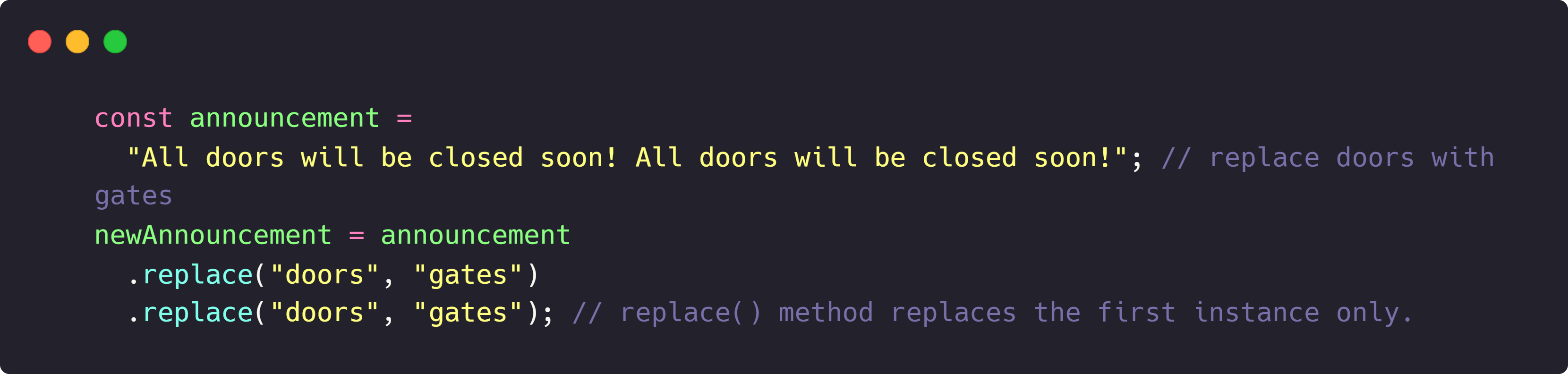
We can also use .replaceAll().
Booleans - These methods returns
booleans.
.includes()
.startsWith()
.endsWith()
Example -
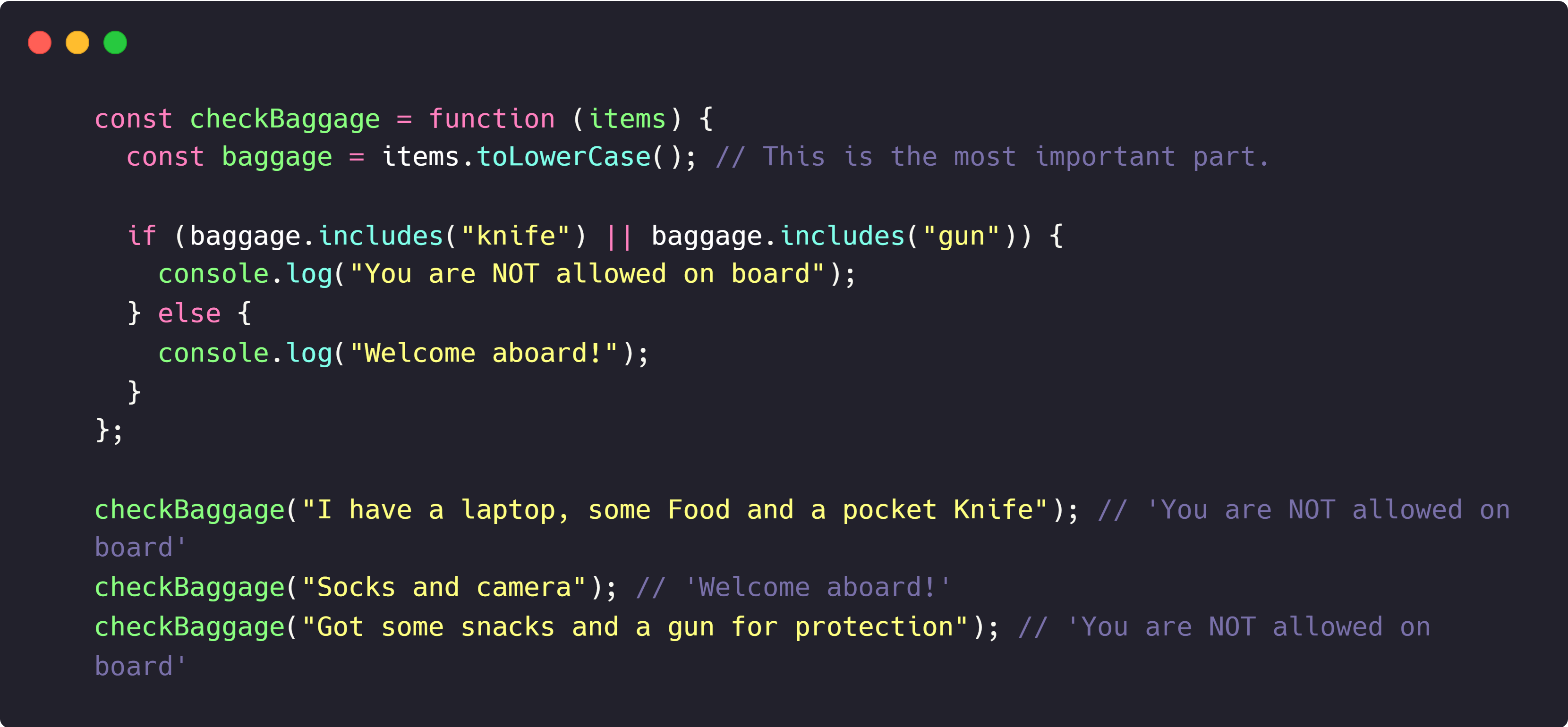
Split and join
split() is to split a
big string into array elements on the basis of a separator and
vice-versa for join().
Example -
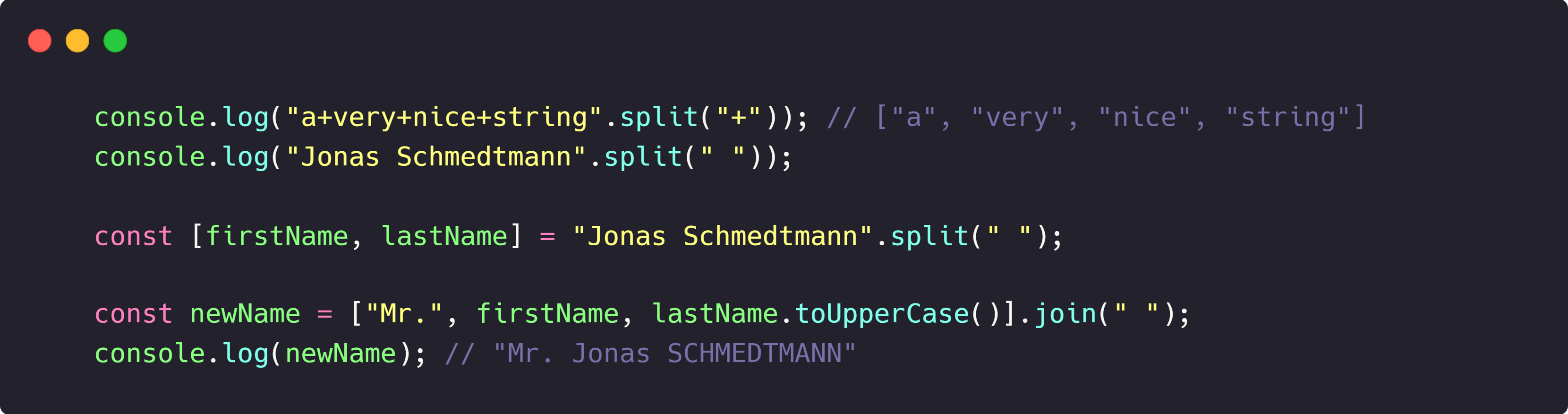
Example - We want to capitalize the name passsed into the function.
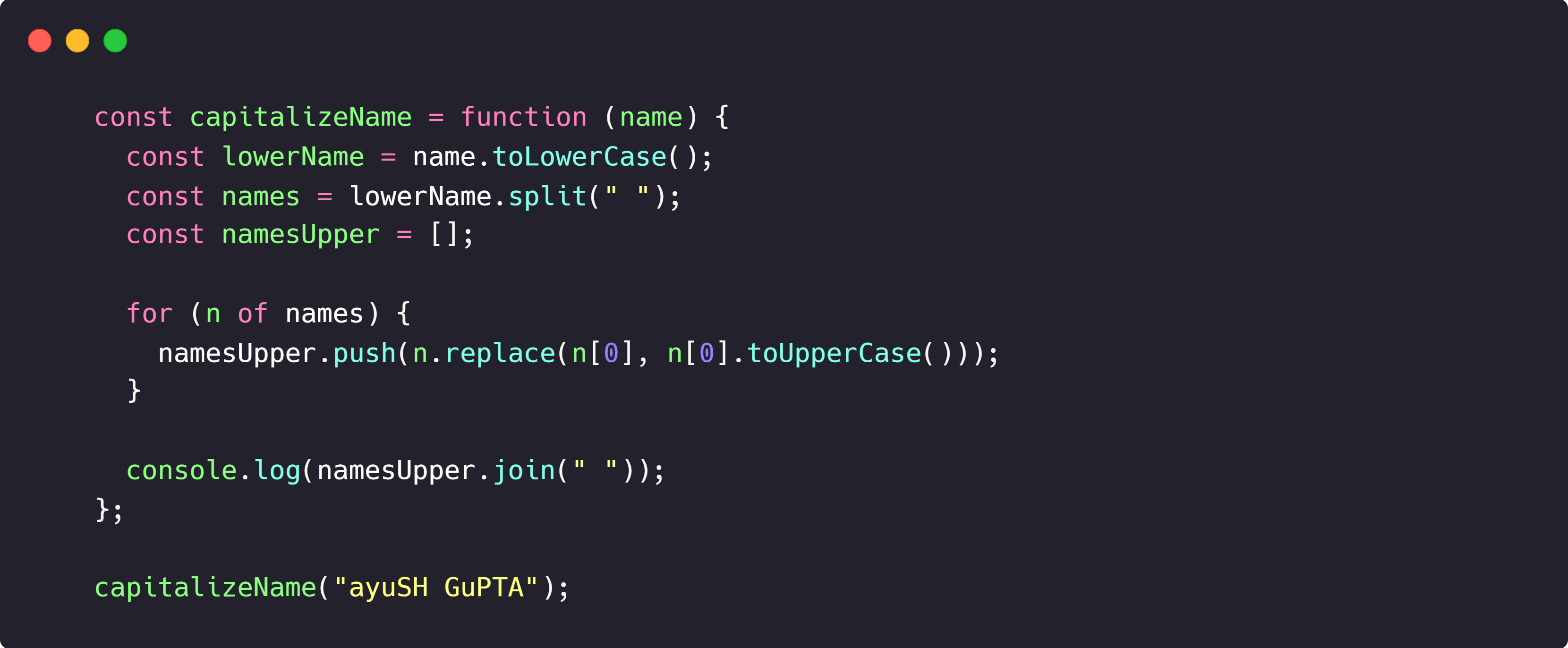
Padding - This method adds a specific
character to the string.
"string".padStart(final length of the
string, Character to be added)
Example -

"string".padEnd(final length of the string, Character to be added)
Example -
