ASYNC JS, AJAX and API
Synchronous code -
Code that is executed line by line, each line of code waits for the
previous code to finish. Blocks code execution.
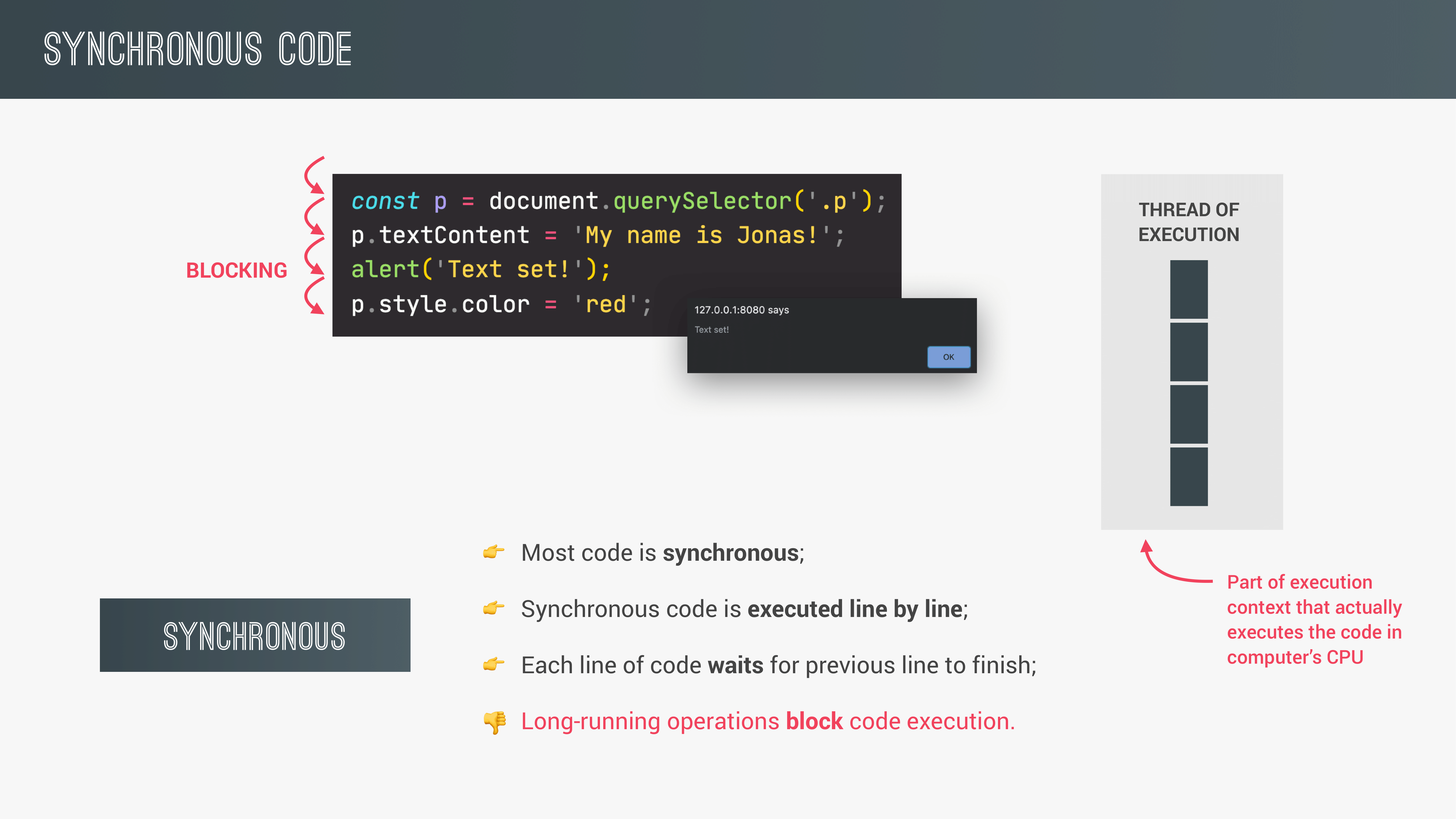
Asynchronous code -
Blocking code gets executed in the BACKGROUND, does not block code
execution. Also called non-blocking code.
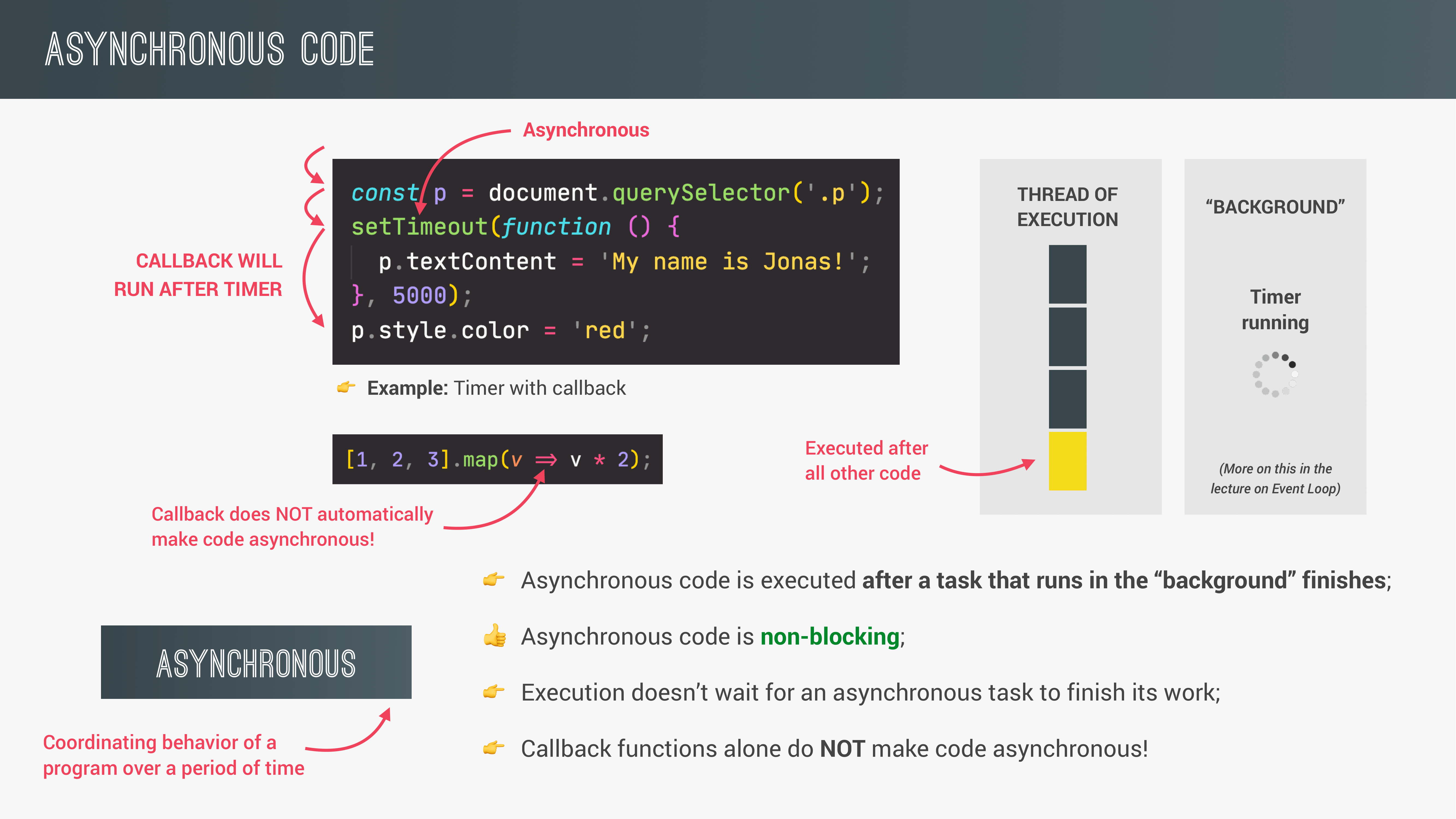
AJAX: Asynchronous JavaScript And XML -
Allows us to communicate with remote web servers in an asynchronous
way. With AJAX calls, we can request data from servers dynamically,
without reloading the page.
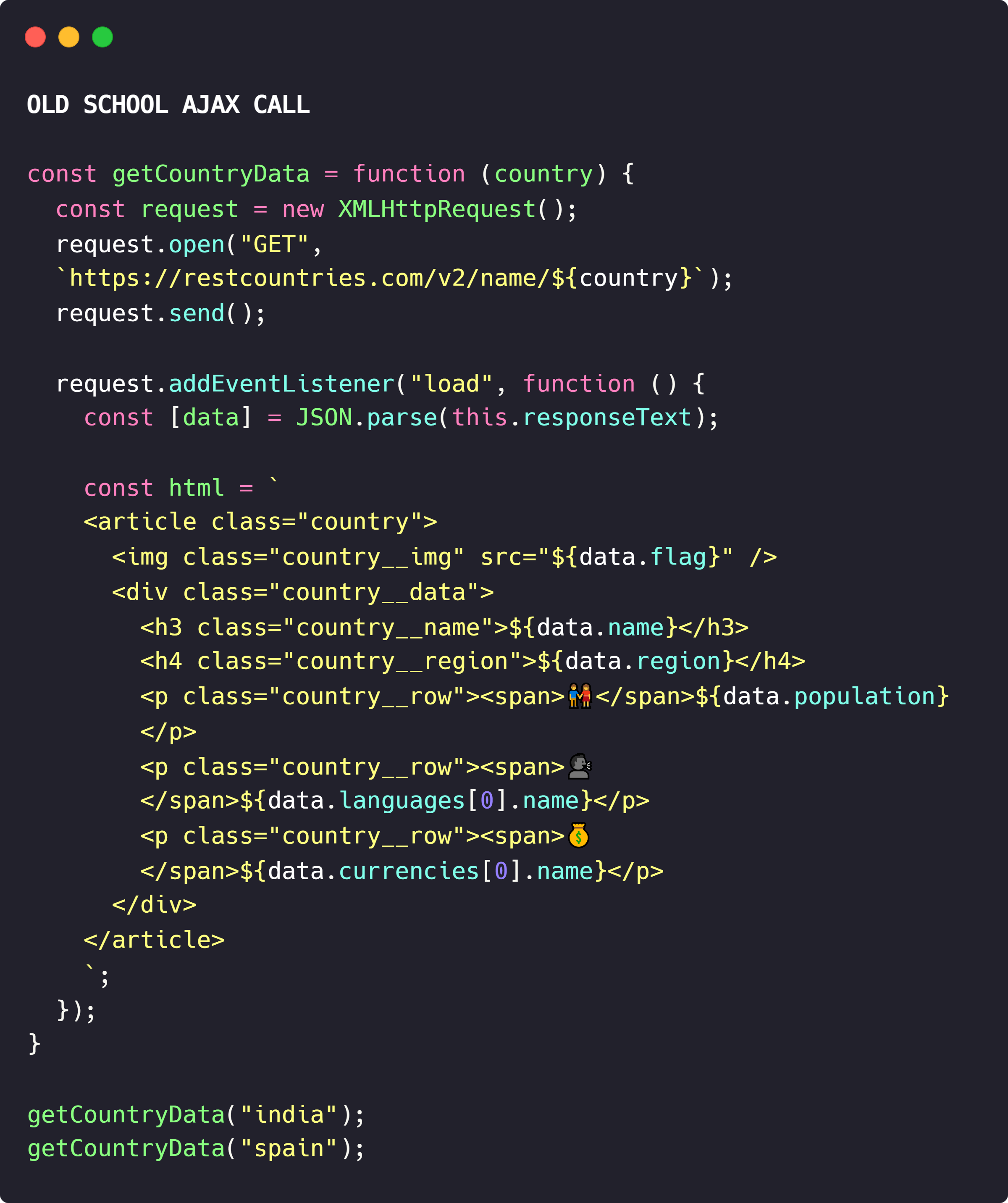
CALLBACK HELL
Callback functions inside callback functions.
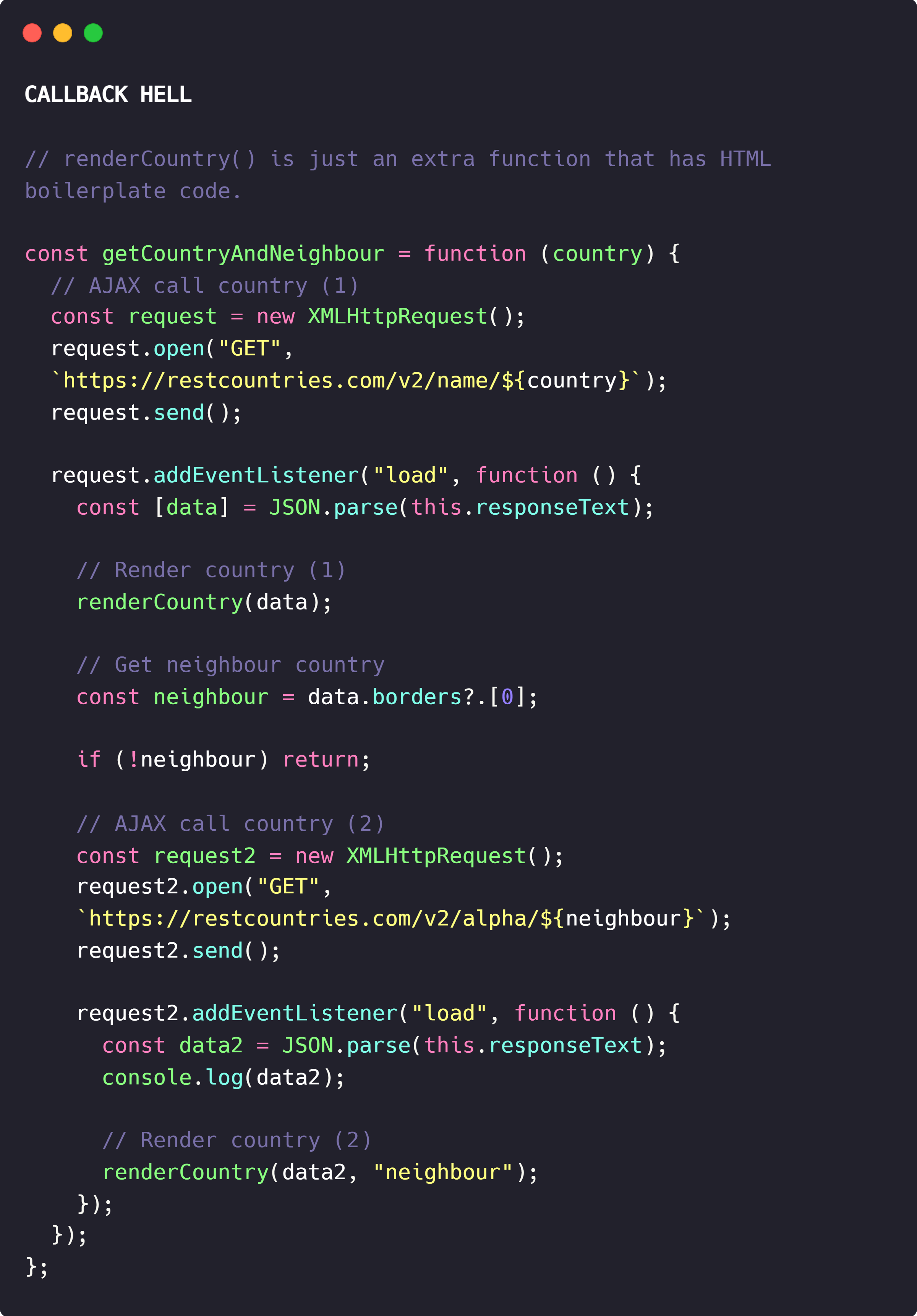
PROMISES AND FETCH API
Promise is an object that is used as a placeholder for the future
result of an asynchronous operation. A promise is like a lottery
ticket.
Fetch is the mordern way of making AJAX calls.
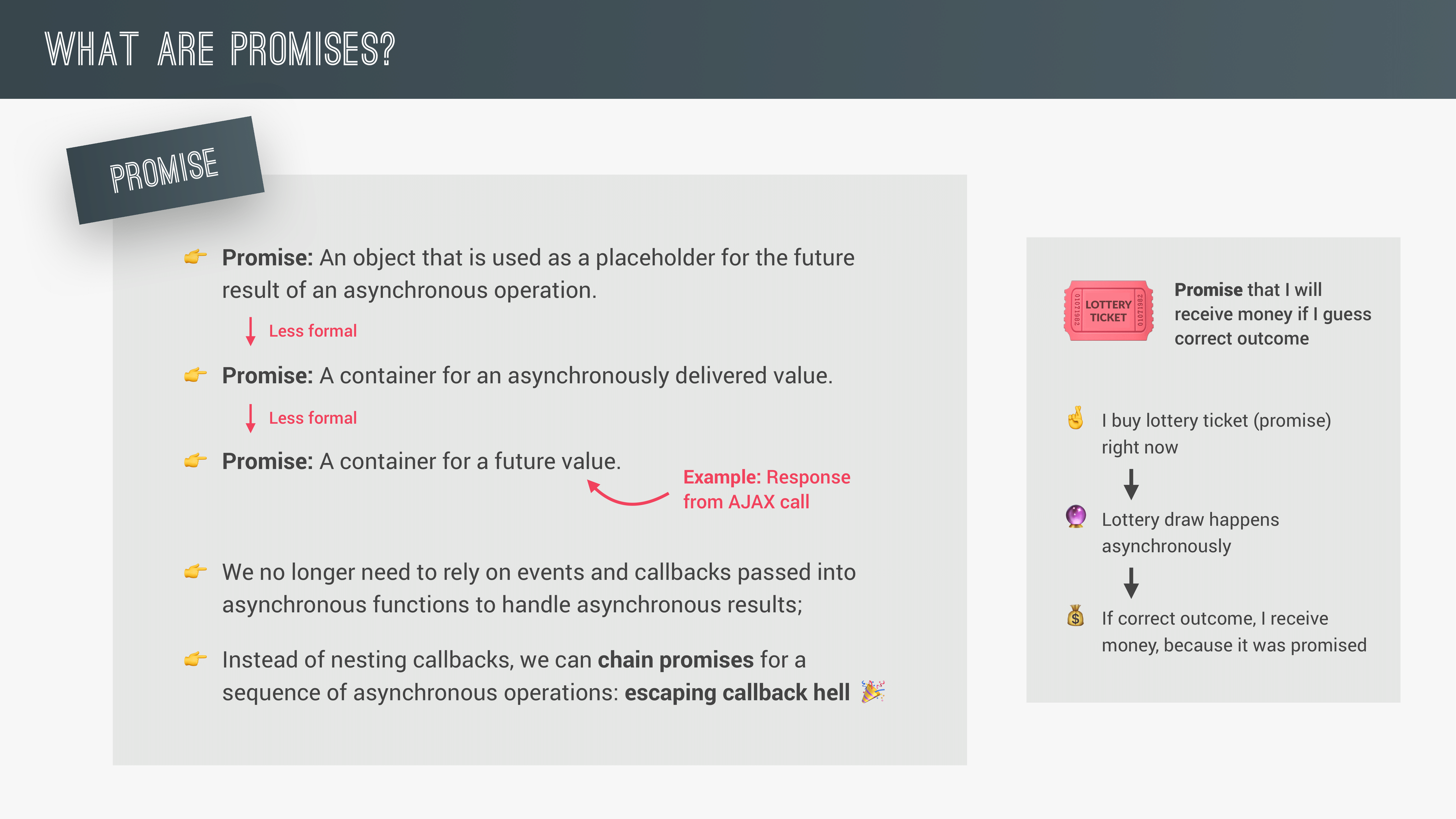
const request = fetch("https://restcountries.com/v2/name/usa"); //
Here, on console logging request, what we get is the promise.
In the beginning, when any value from the asynchronous task is
unavailable, we say that a promise is pending. Now, during this
time, the asynchronous task is still doing its work in the
background. When the asynchronous task finally finishes, we say that
the promise is settled and there are two different types of settled
promises and that's fulfilled promises and rejected promises. A
fulfilled promise is a promise that has successfully resulted in a
value just as we expect it and it's now available to being used. On
the other hand, a rejected promise means that there has been an
error during the asynchronous task.
A promise is either fulfilled or rejected, it is impossible to
change it's state.
Life-cycle of a promise -
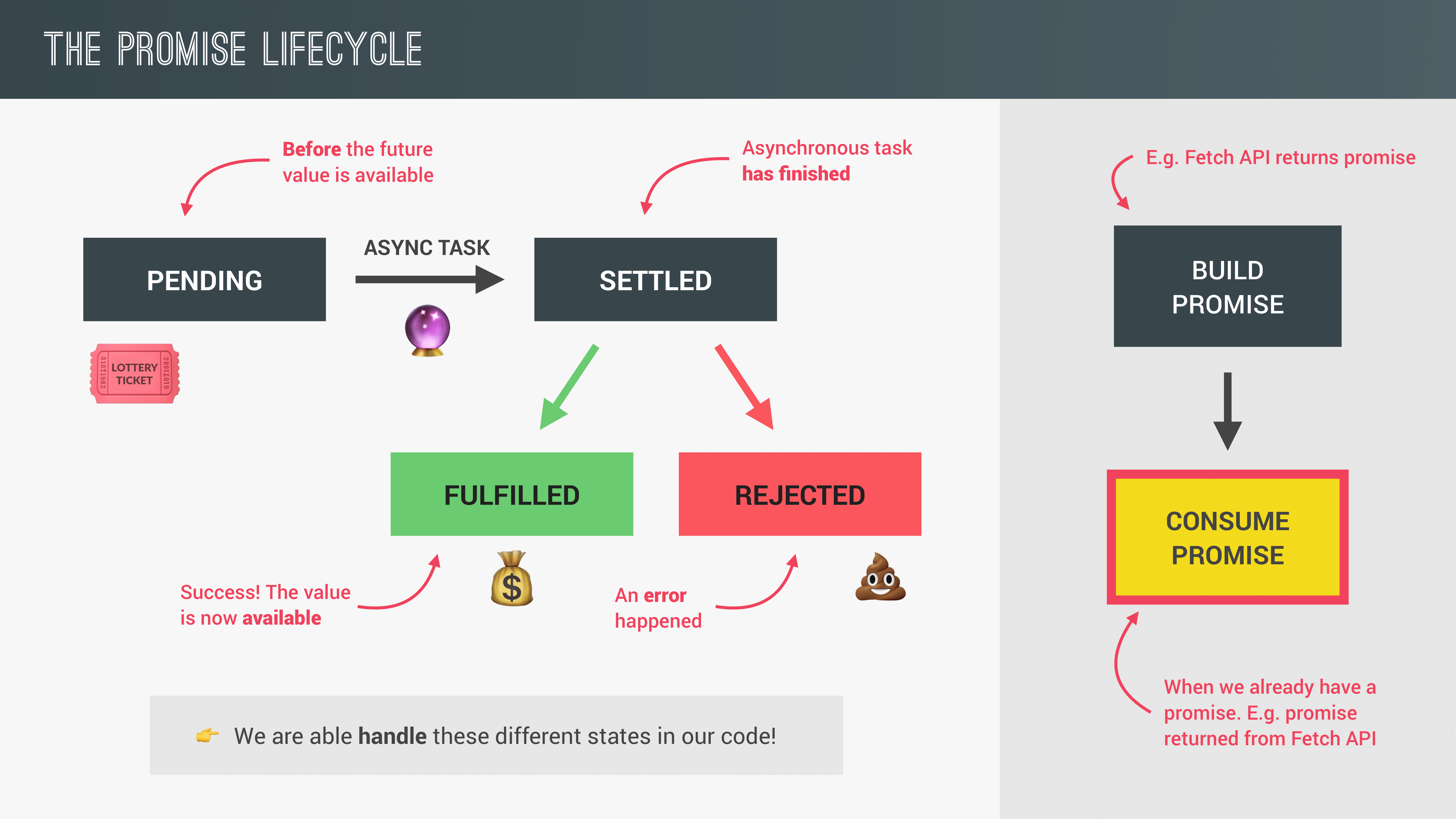
CONSUMING PROMISES
.then() is a method that is available on all the promises.
It's callback function is executed as soon as promise is fulfilled.
It's callback function's argument is the fulfilled value of the
promise that we are calling it upon.
The "then" method always returns a promise, no matter if we actually
return anything or not. But if we do return a value, then that value
will become the fulfillment value of the return promise.
In order to read the body of the response, we need to call .json()
method on it.
json() method is also an asynchronous function, therefore we again
get a new promise, hence we use the .then() method twice in the
example below.
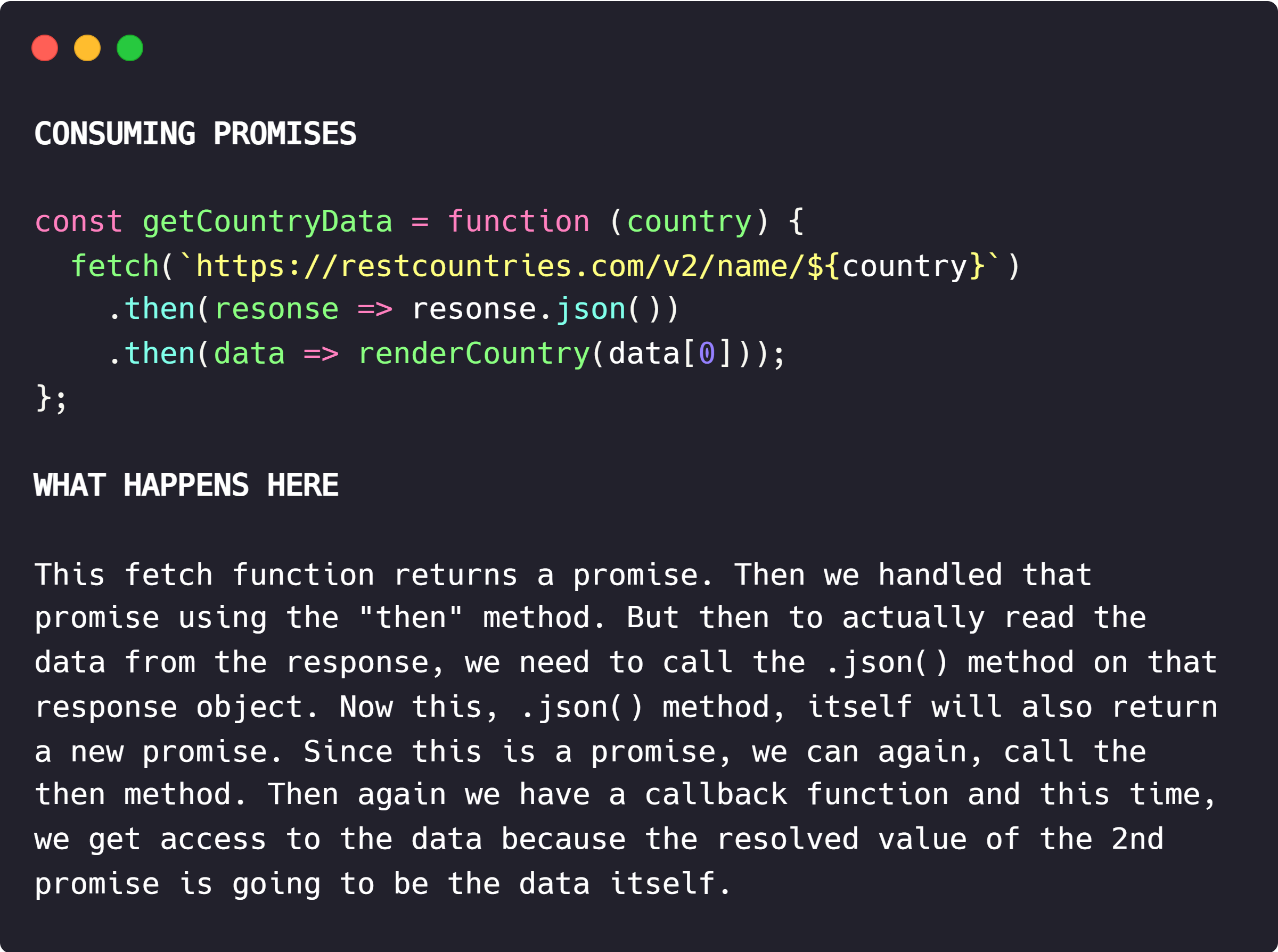