SLICE METHOD
Slices the array into different parts according to input parameters.
Returns a new array.
Excludes the ending element.
SYNTAX - arrayName.slice(startingElement,
endingElement);
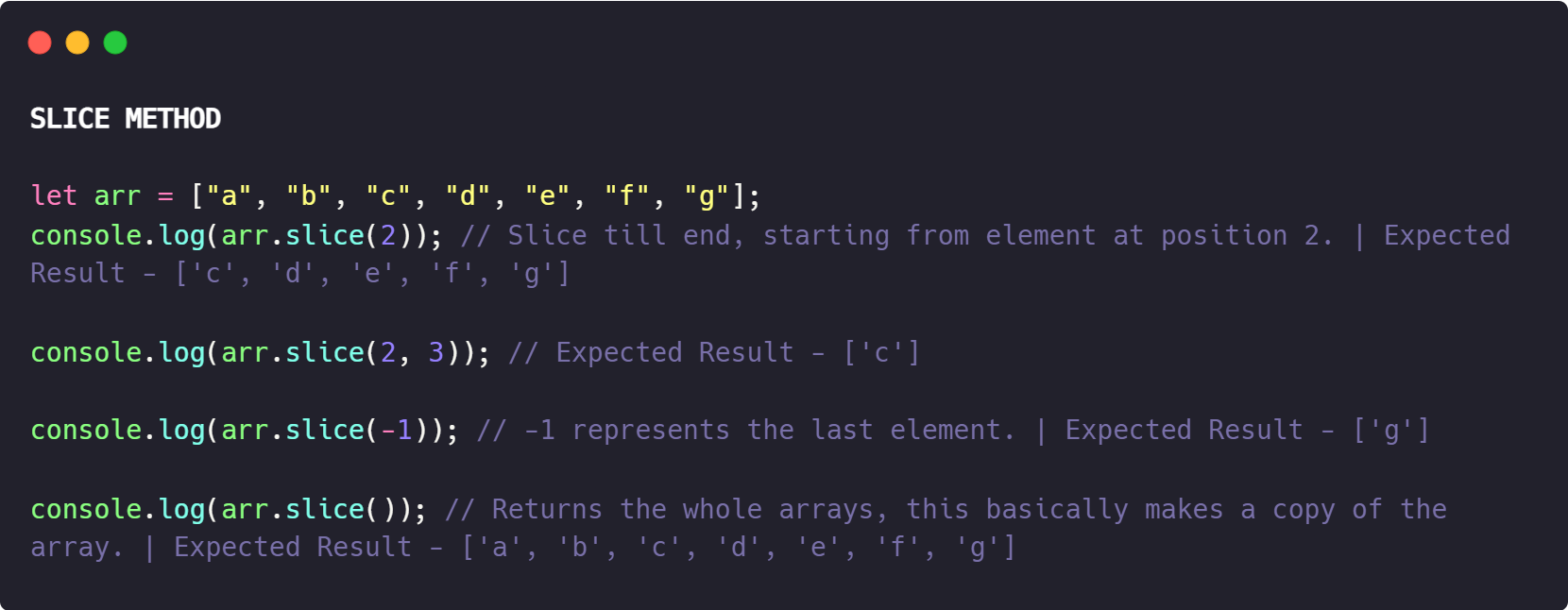
SPLICE METHOD
Same as slice()
This mutates the original array and removes the elements that are
mentioned in splice method.
Returns the removed elements.
Here, the second argument defines the number of elements to be
removed (deleteCount).
SYNTAX - arrayName.splice(startingElement,
deleteCount);
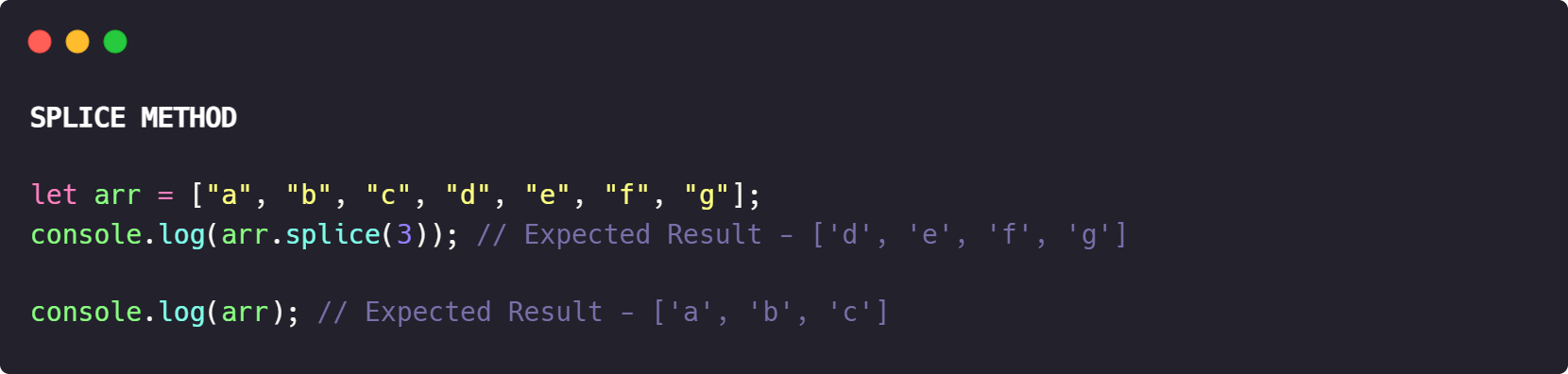
REVERSE METHOD
Reverses the array.
Mutates the original array.
Returns the reversed array.

CONCAT METHOD
Joins 2 arrays.
Does not mutate the original array.
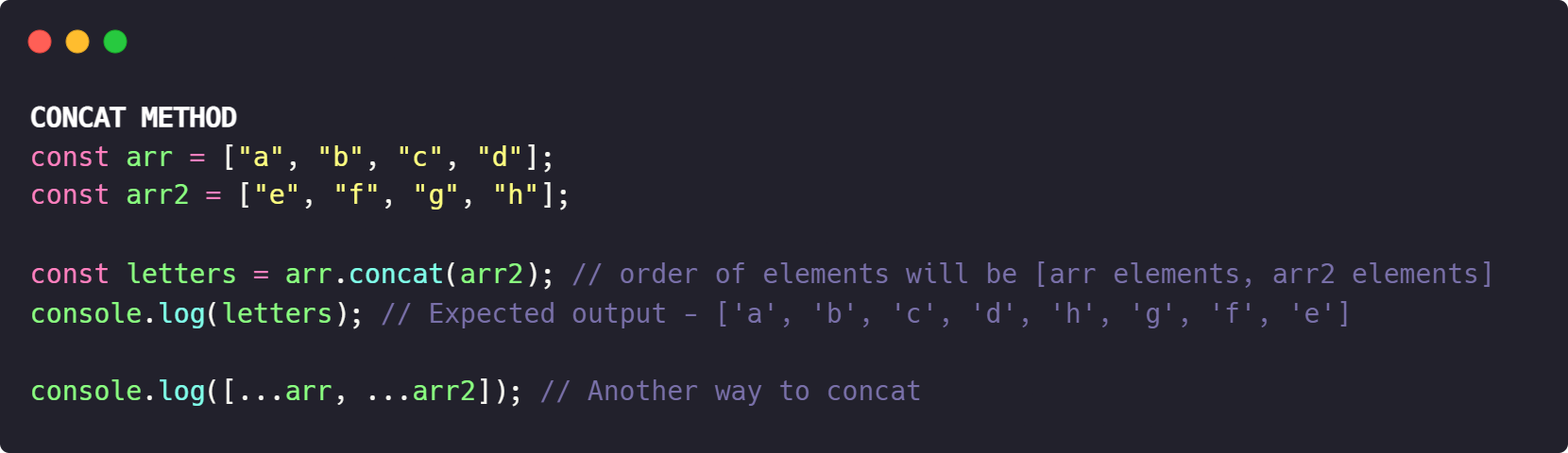
JOIN METHOD
Returns a new string of all the elements joined together by a
defined parameter.
Does not mutate the original array.

FOR OF LOOP
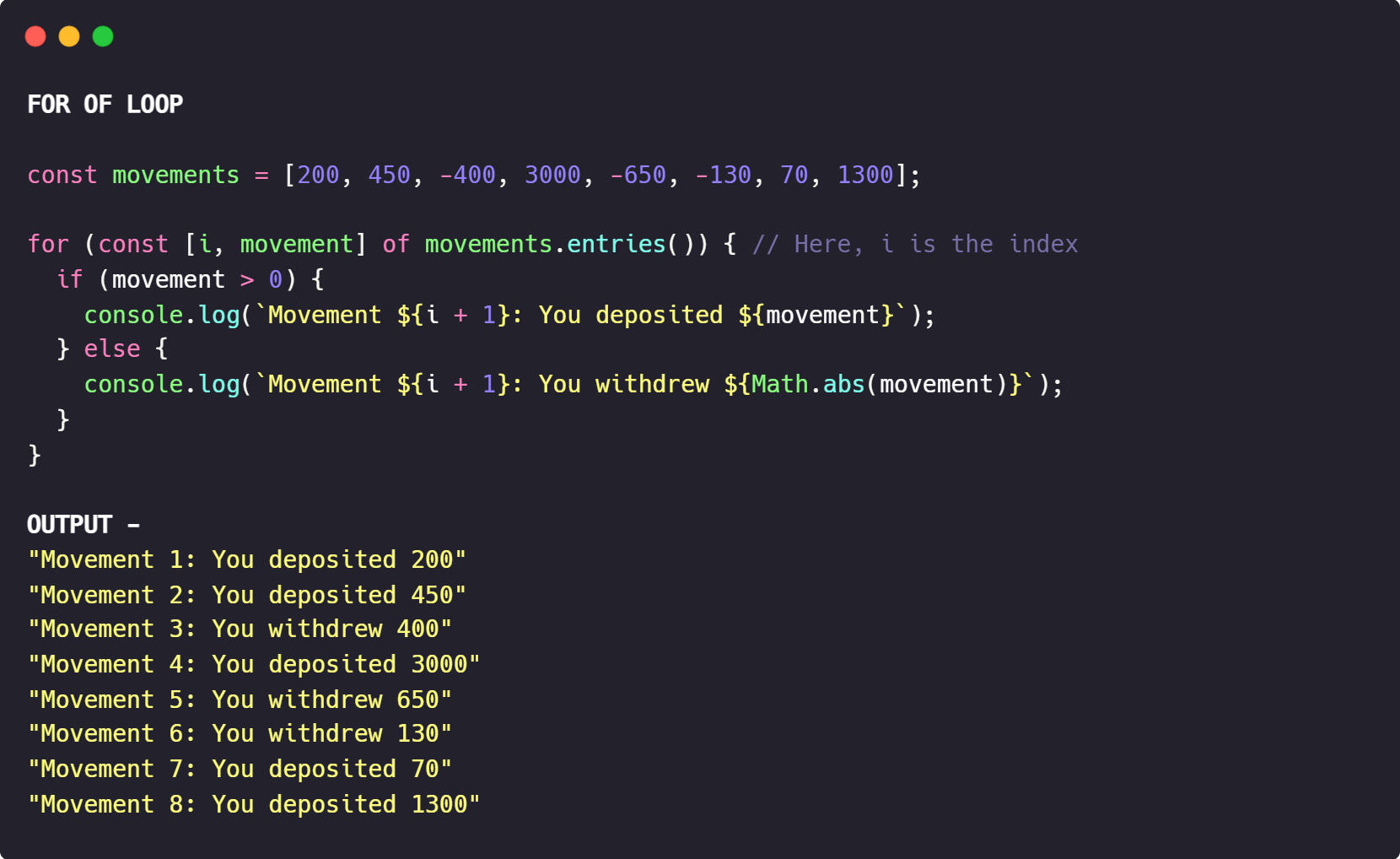
FOR EACH LOOP
The forEach() method calls a function (a callback function) once for
each array element.
Break and continue do not work in forEach loop.
SYNTAX - arrayName.forEach(
function(element, index, the whole array) {})
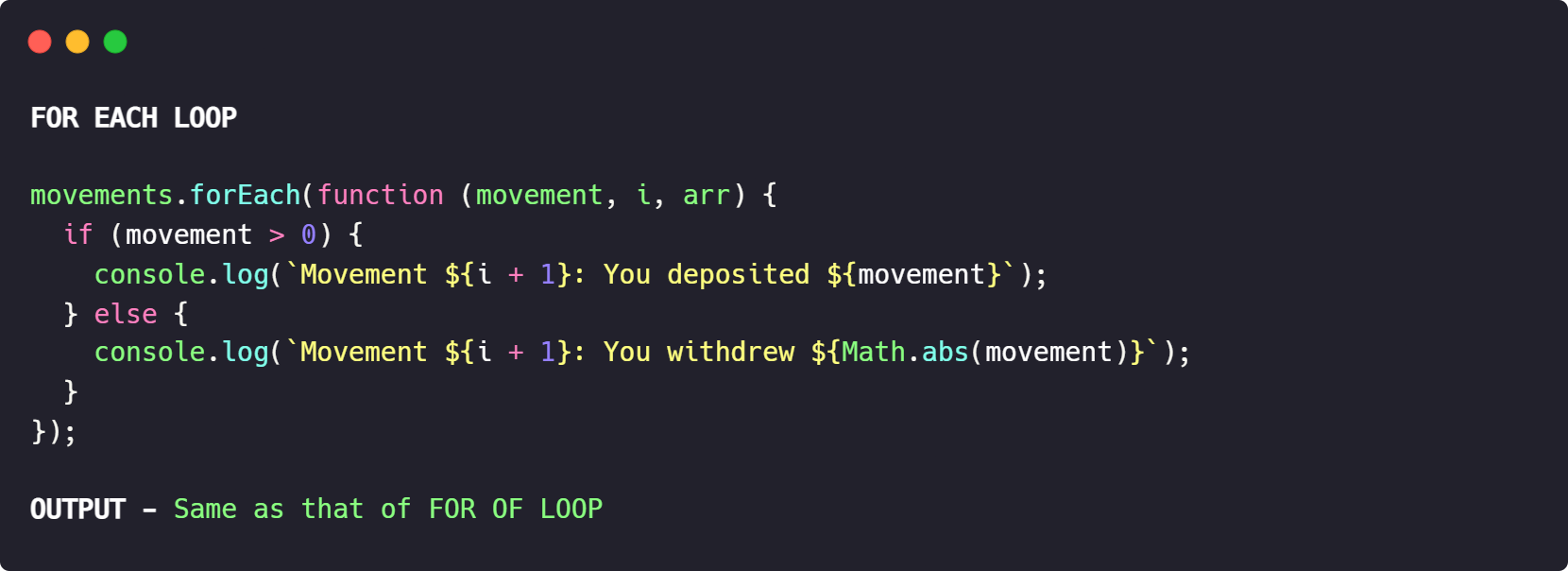
MAP METHOD
Map returns a new array containing the results of, applied
operations on all original array elements.
Does not change the original array.
We can also just use the element parameter.
SYNTAX - array.map( function (element,
index, the whole array){} )
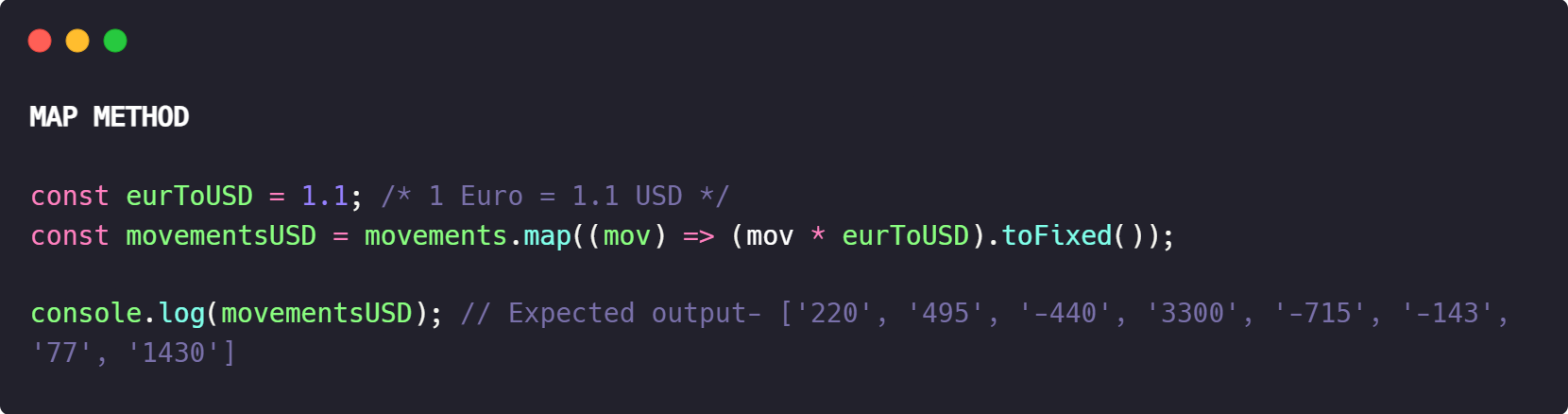
FILTER METHOD
Filter returns a new array containing the array elements that pass a
specified test condition.
SYNTAX - array.filter(function (element,
index of the current element, the whole array))
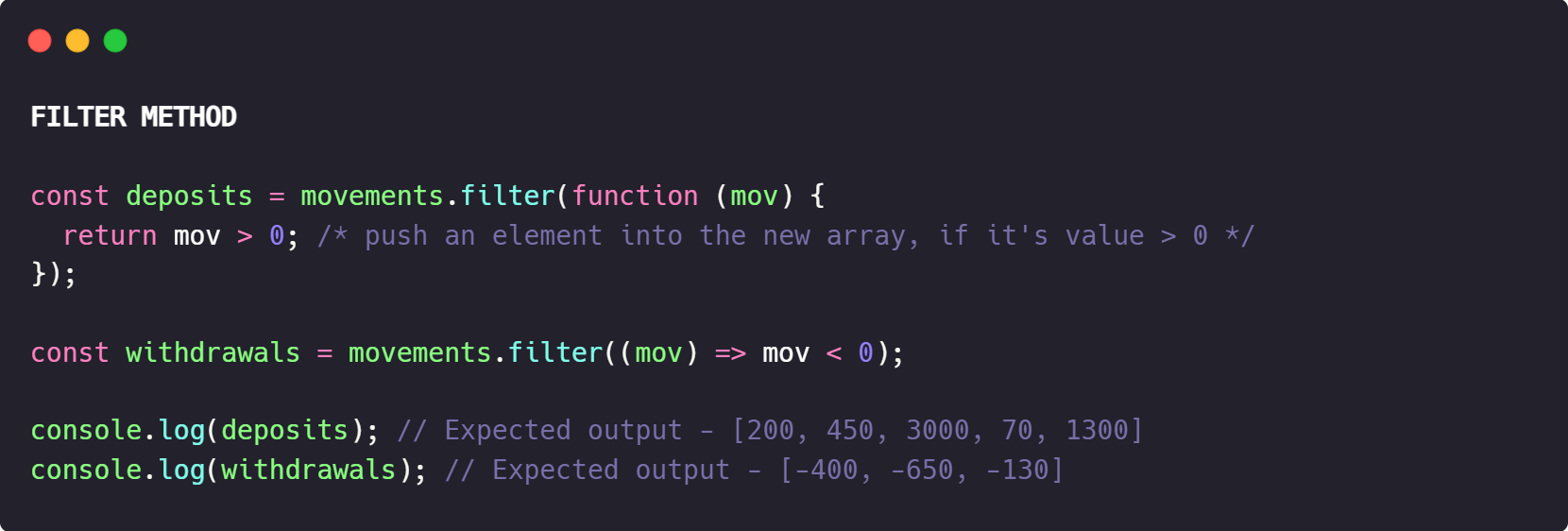
REDUCE METHOD
Reduce boils ("reduces") all array elements down to one single value
and returns that value.
accumulator -> SNOWBALL -> It is the value that is RETURNED in the
last iteration.
SYNTAX - array.reduce(function
(accumulator, current element, index of current element, whole
array) {}, initial value of the accumulator)
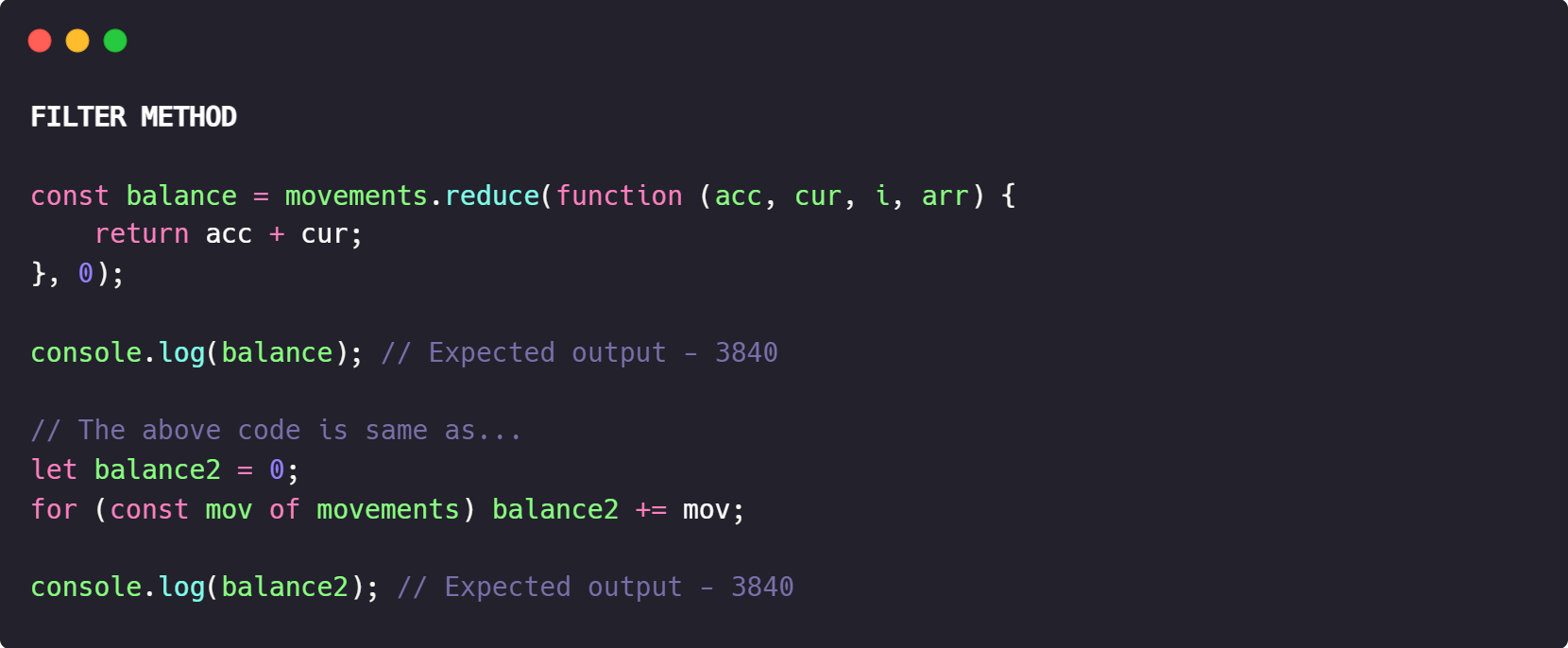
Another example -
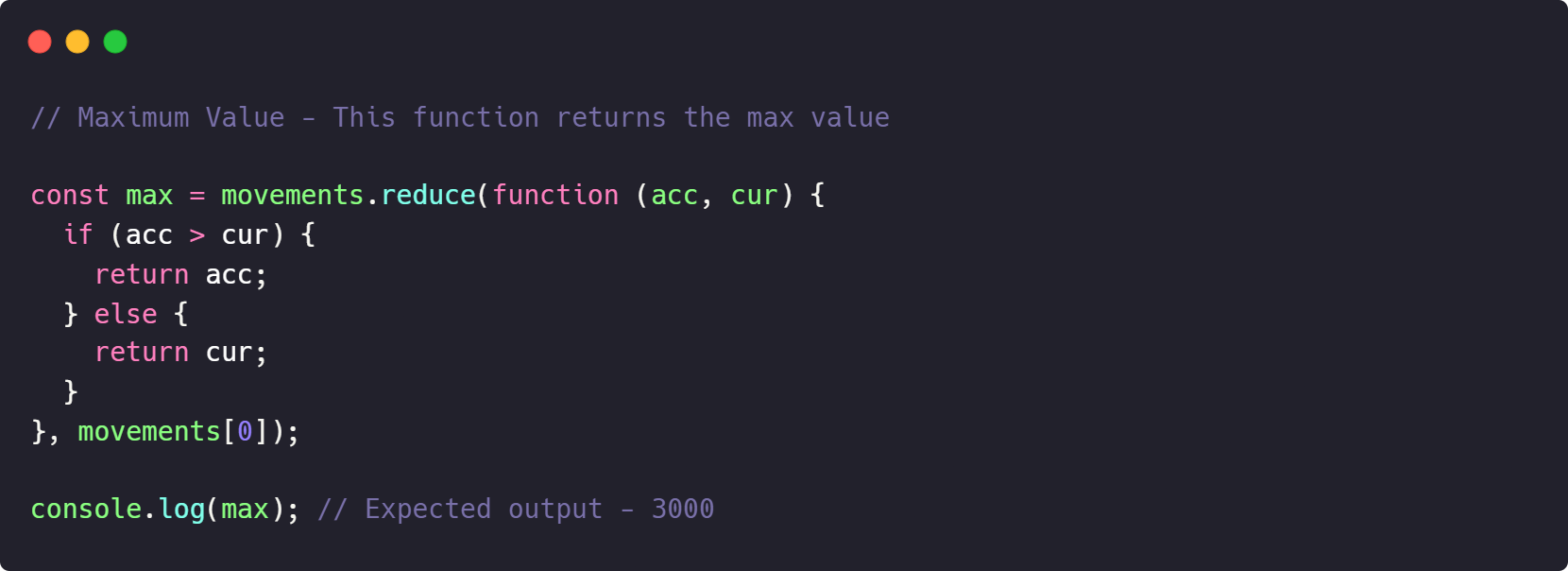
CHAINING DIFFERENT METHODS
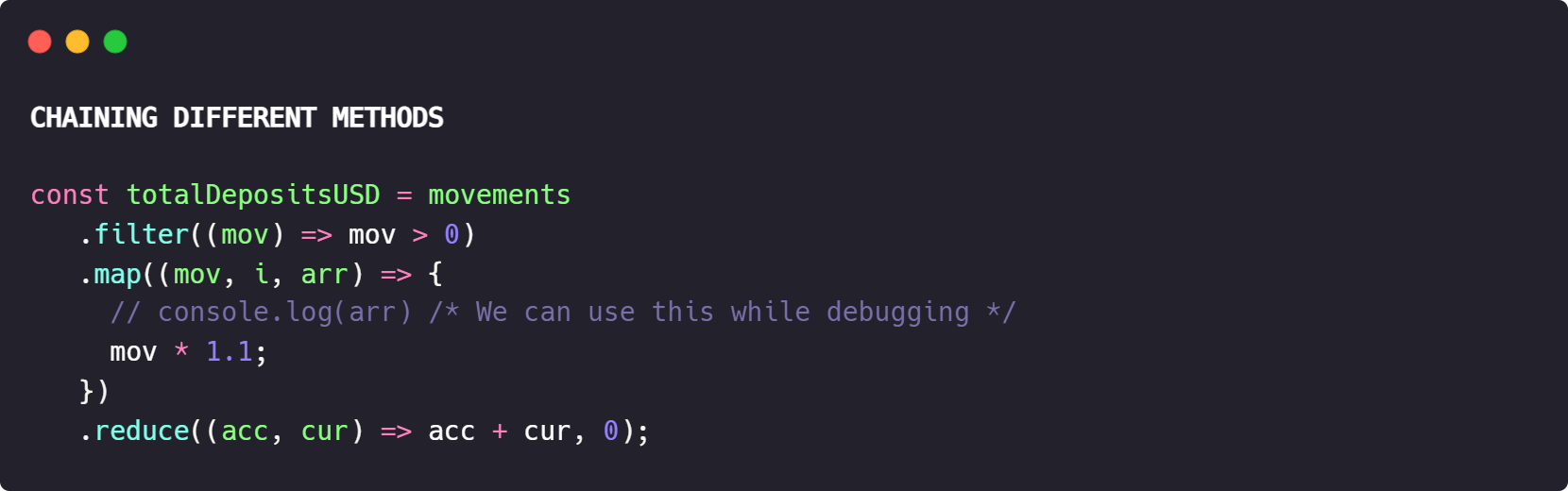
FILTER METHOD
This returns the first element of the array that satisfies the
specified condition.
Diff b/w filter and find is that filter returns an array of all the
elements that satisfies the given condition, while find returns just
the first element.
Returns undefined if no such property is found.
SYNTAX - array.find(function (current
element, index of current element, whole array) {})
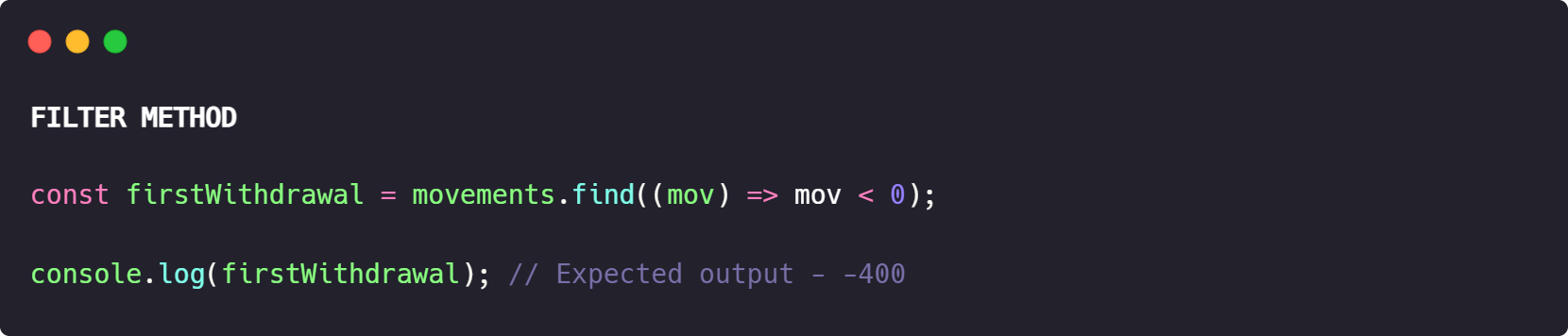
Find method is really useful with arrays of objects as we can find an object (array element) with its property value. EXAMPLE -
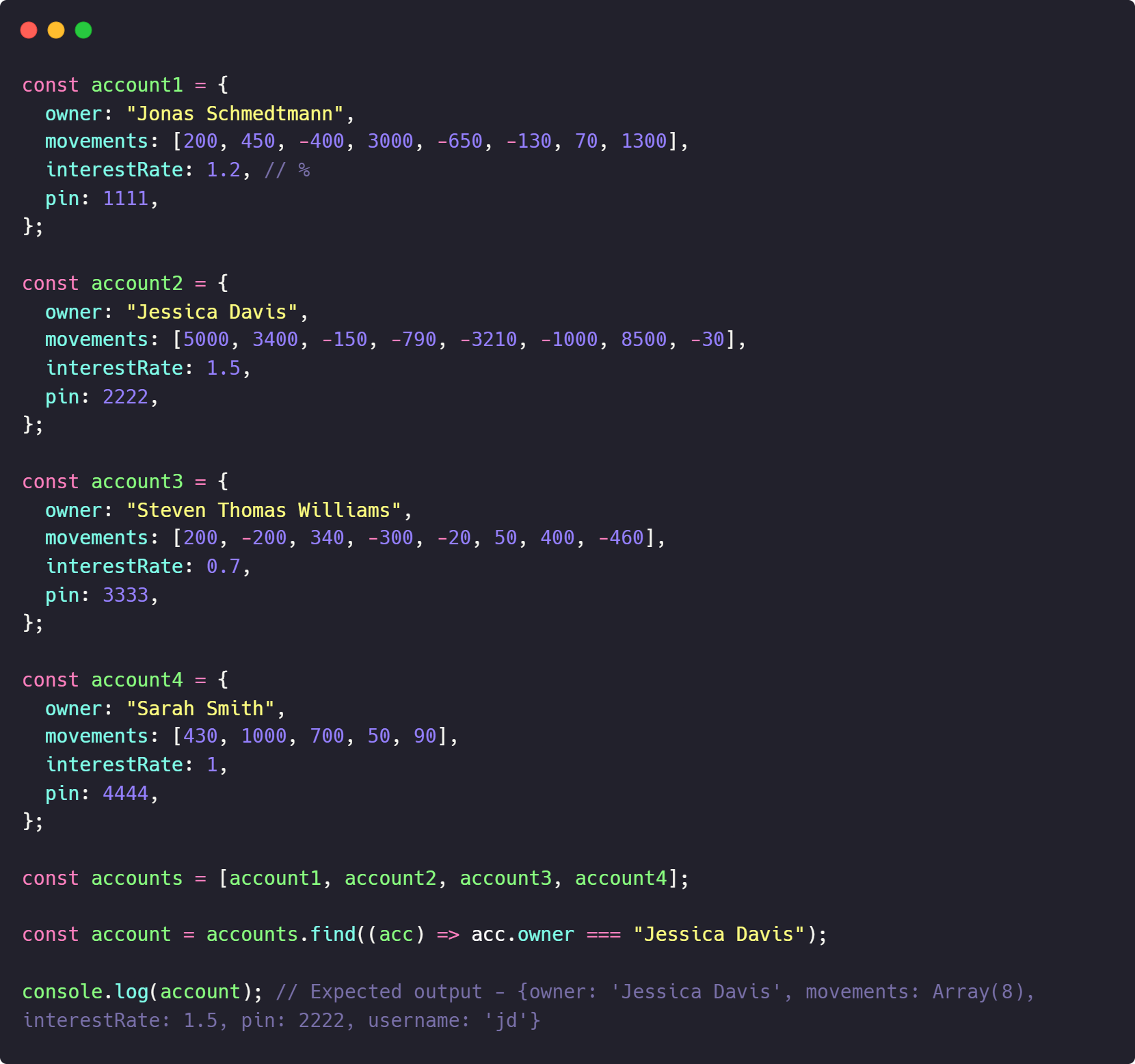